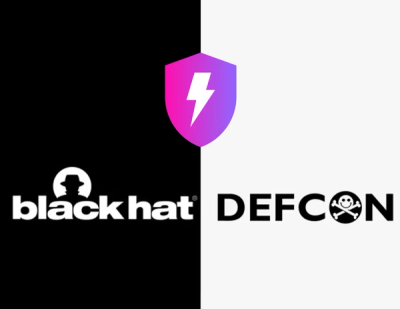
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
github.com/phst/emacs
This package implements Go bindings for the GNU Emacs module API.
This is not an officially supported Google product.
See the package documentation.
The package assumes that ptrdiff_t
and intmax_t
have the same domain as
int64_t
. It also assumes that uintptr_t
has the same domain as uint64_t
.
The C code uses static_assert
to check these assumptions. The C standard
guarantees that int64_t
and
uint64_t
have exactly 64 bits without padding bits and that they use two’s
complement representation. The corresponding Go types int64
and uint64
have
the same representation. This means
that converting int64_t
and uint64_t
to and from the Go types can never lose
data.
The package requires at least Emacs 29. It provides optional support for some Emacs 30 features. Such optional features always require two additional checks:
// Check that emacs-module.h has static support for Emacs 30.
#if defined EMACS_MAJOR_VERSION && EMACS_MAJOR_VERSION >= 30
// Make sure the cast below doesn’t lose information.
static_assert(SIZE_MAX >= PTRDIFF_MAX, "unsupported architecture");
// Check that the Emacs that has loaded this module supports the function.
if ((size_t)env->size > offsetof(emacs_env, function)) {
return env->function(env, …);
}
#endif
// Some workaround in case Emacs 30 isn’t available.
CGo doesn’t support calling C function
pointers. Therefore, the
code wraps all function pointers in the emacs_runtime
and emacs_env
structures in ordinary wrapper functions. The wrapper functions use only the
int64_t
and uint64_t
types in their interfaces.
The package uses a global registry to identify exported functions.
You can compile the examples in the test files to form an example module. In
addition to the examples, this also needs the file example_test.go
to
initialize the examples. The Emacs Lisp file test.el
runs the examples as
ERT
tests.
To build and run all tests, install Bazel and run
bazel test //...
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.