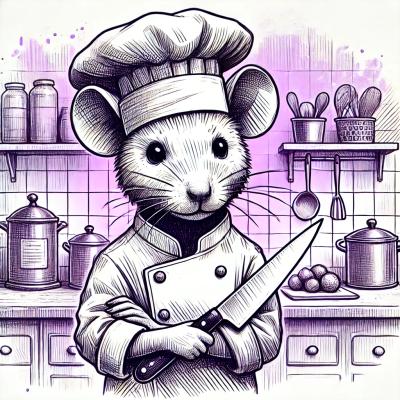
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
git.sr.ht/~kyoto-framework/zen
Zen is an utility-first package that provides a set of commonly used functions, helpers and extensions.
Most of the functions are adapted to be used with html/template
.
We had too many cases of copy-pasting the same code between projects over and over again. So I decided to combine it all in one place.
Code is well-documented, so it's pretty comfortable to use documentation provided by pkg.go.dev
Provided examples doesn't cover all the features of the library. Please refer to the documentation for details.
package main
import (
"time"
"git.sr.ht/~kyoto-framework/zen"
)
// Example of async function
func foo() zen.Future[string] {
return zen.Async(func() (string, error) {
// Imitate work
time.Sleep(time.Second)
// Return result
return "bar", nil
})
}
func main() {
// Sample slice with zen.Range
slice := zen.Range(1, 5) // []int{1, 2, 3, 4, 5}
// Aggregatives
zen.Min(slice) // 1
zen.Max(slice) // 5
zen.Avg(slice) // 3
// Range operations
zen.Filter(slice, func(v int) bool { return v < 3 }) // []int{1, 2}
zen.Map(slice, func(v int) int { return v * 2 }) // []int{2, 4, 6, 8, 10}
zen.In(1, slice) // true
zen.Pop(slice, 1) // ([]int{1, 3, 4, 5}, 2)
zen.Insert(slice, 1, 2) // []int{1, 2, 2, 3, 4, 5}
zen.Last(slice) // 5
zen.Any(slice, func(v int) bool { return v == 2 }) // true
zen.All(slice, func(v int) bool { return v < 6 }) // true
// Inline transformations
zen.Ptr(1) // *int{1} Inline pointer
zen.Bool(3) // bool{true}
zen.Int("5") // int{5}
zen.Float64("6.5") // float64{6.5}
zen.String(7) // string{"7"}
// Map composition (useful for templates)
zen.Compose("foo", 1, "bar", "2") // map[any]any{"foo": 1, "bar": "2"}
// Must for opinionated panic handling
zen.Must(strconv.Atoi("1")) // int{1}, without error
// Await example
futureFoo := foo() // It's not blocking
println("foo call is not blocked")
// Wait for result
result, _ := zen.Await(futureFoo)
println(result)
}
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.