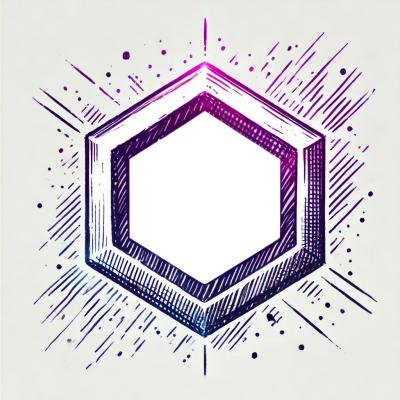
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
github.com/JasonkayZK/consistent-hashing-demo
A simple demo of consistent hashing.
These features have been implemented:
First, start the proxy server:
$ go run main.go
start proxy server: 18888
This will start the proxy server on port 18888.
The server will listen for incoming registration and will forward key searching to the servers.
Then, start the k-v server:
$ go run server/main.go
start server: 8080
Also, the server will register to the proxy server:
register host: localhost:8080 success
Notice: you can register multiple servers to the proxy server:
go run server/main.go -p 8081 go run server/main.go -p 8082 ……
Use curl
to get the key from proxy:
$ curl localhost:18888/key?key=123
key: 123, val: hello: 123
If you query the key at the first time,the key will be cached on the corresponding server for 10s.
The log for proxy server:
Response from host localhost:8080: hello: 123
The log for k-v server:
cached key: {123: hello: 123}
removed cached key after 3s: {123: hello: 123}
You can try to query different key, to check whether they are cached on the different servers:
Response from host localhost:8082: hello: 45363456
Response from host localhost:8080: hello: 4
Response from host localhost:8082: hello: 1
Response from host localhost:8080: hello: 2
Response from host localhost:8082: hello: 3
Response from host localhost:8080: hello: 4
Response from host localhost:8082: hello: 5
Response from host localhost:8080: hello: 6
Response from host localhost:8082: hello: sdkbnfoerwtnbre
Response from host localhost:8082: hello: sd45555254tg423i5gvj4v5
Response from host localhost:8081: hello: 0
Response from host localhost:8082: hello: 032452345
You can request for localhost:18888/key_least
for Load Bound testing:
$ curl localhost:18888/key_least?key=123
key: 123, val: hello: 123
the result is shown below:
start proxy server: 18888
register host: localhost:8080 success
register host: localhost:8081 success
register host: localhost:8082 success
Response from host localhost:8080: hello: 123
Response from host localhost:8080: hello: 123
Response from host localhost:8082: hello: 123
Response from host localhost:8082: hello: 123
Response from host localhost:8081: hello: 123
Response from host localhost:8080: hello: 123
Response from host localhost:8082: hello: 123
Response from host localhost:8081: hello: 123
Response from host localhost:8080: hello: 123
Response from host localhost:8082: hello: 123
Response from host localhost:8081: hello: 123
Response from host localhost:8080: hello: 123
Response from host localhost:8082: hello: 123
Response from host localhost:8081: hello: 123
Response from host localhost:8080: hello: 123
Response from host localhost:8080: hello: 123
Response from host localhost:8082: hello: 123
Response from host localhost:8080: hello: 123
Response from host localhost:8082: hello: 123
Response from host localhost:8082: hello: 123
You can see that, Consistent Hash with Load Bound is far more average!
You can change the
loadBoundFactor
for more experiments:
var ( // the default number of replicas defaultReplicaNum = 10 // the load bound factor // ref: https://research.googleblog.com/2017/04/consistent-hashing-with-bounded-loads.html loadBoundFactor = 0.25 ...... )
Reference:
Linked Blog:
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.