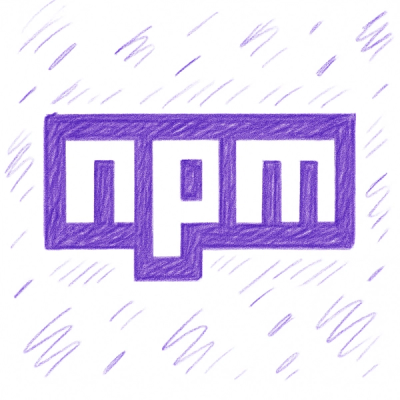
Security News
npm Adopts OIDC for Trusted Publishing in CI/CD Workflows
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
github.com/TouK/http-mock-server
Http Mock Server allows to mock HTTP request using groovy closures.
cd mockserver
mvn clean package assembly:single
java -jar mockserver-full.jar [PORT] [CONFIGURATION_FILE]
Default port is 9999.
If configuration file is passed then port must be defined.
Configuration file is groovy configuration script e.g. :
testRest2 {
port=9998
response='{ req -> \'<response/>\' }'
responseHeaders='{ _ -> [a: "b"] }'
path='testEndpoint'
predicate='{ req -> req.xml.name() == \'request1\'}'
name='testRest2'
}
testRest4 {
soap=true
port=9999
path='testEndpoint'
name='testRest4'
method='PUT'
statusCode=204
}
testRest3 {
port=9999
path='testEndpoint2'
name='testRest3'
}
testRest6 {
port=9999
path='testEndpoint2'
name='testRest6'
maxUses=1
cyclic=true
}
testRest {
imports {
aaa='bbb'
ccc='bla'
}
port=10001
path='testEndpoint'
name='testRest'
}
testHttps {
soap=false
port=10443
path='testHttps'
name='testHttps'
method='GET'
https={
keystorePath='/tmp/keystore.jks'
keystorePassword='keystorePass'
keyPassword='keyPass'
truststorePath='/tmp/truststore.jks'
truststorePassword='truststorePass'
requireClientAuth=true
}
}
Docker and docker-compose is needed.
./buildImage.sh
docker-compose up -d
Built image is available at https://hub.docker.com/r/alien11689/mockserver/
RemoteMockServer remoteMockServer = new RemoteMockServer('localhost', <PORT>)
remoteMockServer.addMock(new AddMock(
name: '...',
path: '...',
port: ...,
predicate: '''...''',
response: '''...''',
soap: ...,
statusCode: ...,
method: ...,
responseHeaders: ...,
schema: ...,
maxUses: ...,
cyclic: ...,
https: new Https(
keystorePath: '/tmp/keystore.jks',
keystorePassword: 'keystorePass',
keyPassword: 'keyPass',
truststorePath: '/tmp/truststore.jks',
truststorePassword: 'truststorePass',
requireClientAuth: true
)
))
Send POST request to localhost:/serverControl
<addMock xmlns="http://touk.pl/mockserver/api/request">
<name>...</name>
<path>...</path>
<port>...</port>
<predicate>...</predicate>
<response>...</response>
<soap>...</soap>
<statusCode>...</statusCode>
<method>...</method>
<responseHeaders>...</responseHeaders>
<schema>...</schema>
<imports alias="..." fullClassName="..."/>
<maxUses>...</maxUses>
<cyclic>...</cyclic>
<https>
<keystorePath>/tmp/keystore.jks</keystorePath>
<keystorePassword>keystorePass</keystorePassword>
<keyPassword>keyPass</keyPassword>
<truststorePath>/tmp/truststore.jks</truststorePath>
<truststorePassword>truststorePass</truststorePassword>
<requireClientAuth>true</requireClientAuth>
</https>
</addMock>
POST
, ANY_METHOD
matches all HTTP methodsalias
is the name of fullClassName
available in closure; fullClassName
must be available on classpath of mock servermaxUses
uses at the end of the mock list (by default false)HTTP and HTTPS should be started on separated ports.
In closures input parameter (called req) contains properties:
Response if success:
<mockAdded xmlns="http://touk.pl/mockserver/api/response"/>
Response with error message if failure:
<exceptionOccured xmlns="http://touk.pl/mockserver/api/response">...</exceptionOccured>
Mock could be peeked to get get report of its invocations.
List<MockEvent> mockEvents = remoteMockServer.peekMock('...')
Send POST request to localhost:/serverControl
<peekMock xmlns="http://touk.pl/mockserver/api/request">
<name>...</name>
</peekMock>
Response if success:
<mockPeeked xmlns="http://touk.pl/mockserver/api/response">
<mockEvent>
<request>
<text>...</text>
<headers>
<header name="...">...</header>
...
</headers>
<queryParams>
<queryParam name="...">...</queryParam>
...
</queryParams>
<path>
<pathPart>...</pathPart>
...
</path>
</request>
<response>
<statusCode>...</statusCode>
<text>...</text>
<headers>
<header name="...">...</header>
...
</headers>
</response>
</mockEvent>
</mockPeeked>
Response with error message if failure:
<exceptionOccured xmlns="http://touk.pl/mockserver/api/response">...</exceptionOccured>
When mock was used it could be unregistered by name. It also optionally returns report of mock invocations if second parameter is true.
List<MockEvent> mockEvents = remoteMockServer.removeMock('...', ...)
Send POST request to localhost:/serverControl
<removeMock xmlns="http://touk.pl/mockserver/api/request">
<name>...</name>
<skipReport>...</skipReport>
</removeMock>
Response if success (and skipReport not given or equal false):
<mockRemoved xmlns="http://touk.pl/mockserver/api/response">
<mockEvent>
<request>
<text>...</text>
<headers>
<header name="...">...</header>
...
</headers>
<queryParams>
<queryParam name="...">...</queryParam>
...
</queryParams>
<path>
<pathPart>...</pathPart>
...
</path>
</request>
<response>
<statusCode>...</statusCode>
<text>...</text>
<headers>
<header name="...">...</header>
...
</headers>
</response>
</mockEvent>
</mockRemoved>
If skipReport is set to true then response will be:
<mockRemoved xmlns="http://touk.pl/mockserver/api/response"/>
Response with error message if failure:
<exceptionOccured xmlns="http://touk.pl/mockserver/api/response">...</exceptionOccured>
List<RegisteredMock> mocks = remoteMockServer.listMocks()
Send GET request to localhost:/serverControl
Response:
<mocks>
<mock>
<name>...</name>
<path>...</path>
<port>...</port>
<predicate>...</predicate>
<response>...</response>
<responseHeaders>...</responseHeaders>
<soap>...</soap>
<method>...</method>
<statusCode>...</statusCode>
<imports alias="..." fullClassName="..."/>
</mock>
...
</mocks>
ConfigObject mocks = remoteMockServer.getConfiguration()
Send GET request to localhost:/serverControl/configuration
Response:
testRest2 {
port=9998
response='{ req -> \'<response/>\' }'
responseHeaders='{ _ -> [a: "b"] }'
path='testEndpoint'
predicate='{ req -> req.xml.name() == \'request1\'}'
name='testRest2'
}
testRest4 {
soap=true
port=9999
path='testEndpoint'
name='testRest4'
method='PUT'
statusCode=204
}
testRest3 {
port=9999
path='testEndpoint2'
name='testRest3'
}
testRest6 {
port=9999
path='testEndpoint2'
name='testRest6'
}
testRest {
imports {
aaa='bbb'
ccc='bla'
}
port=10001
path='testEndpoint'
name='testRest'
}
This response could be saved to file and passed as it is during mock server creation.
Mockserver is available at philanthropist.touk.pl
.
Just add repository to maven pom:
<project>
...
<repositories>
...
<repository>
<id>touk</id>
<url>https://philanthropist.touk.pl/nexus/content/repositories/releases</url>
</repository>
...
</repositories>
...
</project>
Q: Can I have two mocks returning responses interchangeably for the same request?
A: Yes, you can. Just set two mocks with maxUses: 1
and cyclic: true
.
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
Research
/Security News
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
Security News
The CNA Scorecard ranks CVE issuers by data completeness, revealing major gaps in patch info and software identifiers across thousands of vulnerabilities.