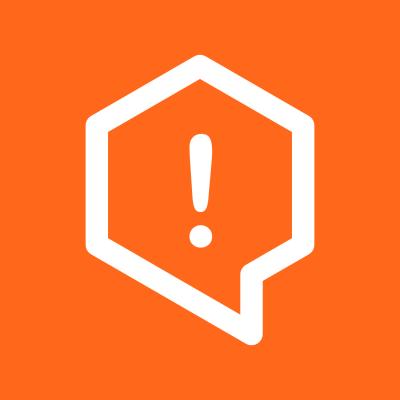
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
github.com/akamai/AkamaiOPEN-edgegrid-golang
This library implements an Authentication handler for net/http that provides the Akamai OPEN Edgegrid Authentication scheme. For more information visit the Akamai OPEN Developer Community. This library has been released as a v1 library though future development will be on the develop branch for version v3.
The v3 version of this module is under active development and provides a subset of Akamai APIs for use in the Akamai Terraform Provider.
New users are encouraged to adopt v3 version it is a simpler API wrapper with little to no business logic.
Current direct users of this v1.x.x library are recommended to continue to use the v1 version as initialization and package structure has significantly changed in v3 and will require substantial work to migrate existing applications. Non-backwards compatible changes were made to improve the code quality and make the project more maintainable.
GET Example:
package main
import (
"fmt"
"io/ioutil"
"github.com/akamai/AkamaiOPEN-edgegrid-golang/client-v1"
"github.com/akamai/AkamaiOPEN-edgegrid-golang/edgegrid"
)
func main() {
config, _ := edgegrid.Init("~/.edgerc", "default")
// Retrieve all locations for diagnostic tools
req, _ := client.NewRequest(config, "GET", "/diagnostic-tools/v2/ghost-locations/available", nil)
resp, _ := client.Do(config, req)
defer resp.Body.Close()
byt, _ := ioutil.ReadAll(resp.Body)
fmt.Println(string(byt))
}
Parameter Example:
package main
import (
"fmt"
"io/ioutil"
"github.com/akamai/AkamaiOPEN-edgegrid-golang/client-v1"
"github.com/akamai/AkamaiOPEN-edgegrid-golang/edgegrid"
)
func main() {
config, _ := edgegrid.Init("~/.edgerc", "default")
// Retrieve dig information for specified location
req, _ := client.NewRequest(config, "GET", "/diagnostic-tools/v2/ghost-locations/zurich-switzerland/dig-info", nil)
q := req.URL.Query()
q.Add("hostName", "developer.akamai.com")
q.Add("queryType", "A")
req.URL.RawQuery = q.Encode()
resp, _ := client.Do(config, req)
defer resp.Body.Close()
byt, _ := ioutil.ReadAll(resp.Body)
fmt.Println(string(byt))
}
POST Example:
package main
import (
"fmt"
"io/ioutil"
"net/http"
"github.com/akamai/AkamaiOPEN-edgegrid-golang/client-v1"
"github.com/akamai/AkamaiOPEN-edgegrid-golang/edgegrid"
)
func main() {
config, _ := edgegrid.Init("~/.edgerc", "default")
// Acknowledge a map
req, _ := client.NewRequest(config, "POST", "/siteshield/v1/maps/1/acknowledge", nil)
resp, _ := client.Do(config, req)
defer resp.Body.Close()
byt, _ := ioutil.ReadAll(resp.Body)
fmt.Println(string(byt))
}
PUT Example:
package main
import (
"fmt"
"io/ioutil"
"net/http"
"github.com/akamai/AkamaiOPEN-edgegrid-golang/client-v1"
"github.com/akamai/AkamaiOPEN-edgegrid-golang/edgegrid"
)
func main() {
config, _ := edgegrid.Init("~/.edgerc", "default")
body := []byte("{\n \"name\": \"Simple List\",\n \"type\": \"IP\",\n \"unique-id\": \"345_BOTLIST\",\n \"list\": [\n \"192.168.0.1\",\n \"192.168.0.2\",\n ],\n \"sync-point\": 0\n}")
// Update a Network List
req, _ := client.NewJSONRequest(config, "PUT", "/network-list/v1/network_lists/unique-id?extended=extended", body)
resp, _ := client.Do(config, req)
defer resp.Body.Close()
byt, _ := ioutil.ReadAll(resp.Body)
fmt.Println(string(byt))
}
Alternatively, your program can read it from config struct.
package main
import (
"fmt"
"io/ioutil"
"net/http"
"github.com/akamai/AkamaiOPEN-edgegrid-golang/client-v1"
"github.com/akamai/AkamaiOPEN-edgegrid-golang/edgegrid"
)
func main() {
config := edgegrid.Config{
Host : "xxxxxx.luna.akamaiapis.net",
ClientToken: "xxxx-xxxxxxxxxxx-xxxxxxxxxxx",
ClientSecret: "xxxxxxxxxxxxxxxxxxxxxxxxxxxxx",
AccessToken: "xxxx-xxxxxxxxxxx-xxxxxxxxxxx",
MaxBody: 1024,
HeaderToSign: []string{
"X-Test1",
"X-Test2",
"X-Test3",
},
Debug: false,
}
// Retrieve all locations for diagnostic tools
req, _ := client.NewRequest(config, "GET", fmt.Sprintf("https://%s/diagnostic-tools/v2/ghost-locations/available",config.Host), nil)
resp, _ := client.Do(config, req)
defer resp.Body.Close()
byt, _ := ioutil.ReadAll(resp.Body)
fmt.Println(string(byt))
}
Davey Shafik - Developer Evangelist @ Akamai Technologies Nick Juettner - Software Engineer @ Zalando SE
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.