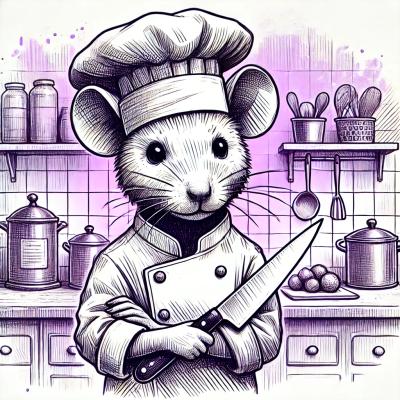
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
github.com/clubnft/scheduler
Go Task scheduler is a small library that you can use within your application that enables you to execute callbacks (goroutines) after a pre-defined amount of time. GTS also provides task storage which is used to invoke callbacks for tasks which couldn't be executed during down-time as well as maintaining a history of the callbacks that got executed.
** Fixed in this fork:
** Features
Installation #+BEGIN_SRC shell go get github.com/ClubNFT/scheduler #+END_SRC
How To Use
Instantiate a scheduler as follows:
#+BEGIN_SRC go s := scheduler.New(storage, funcManager) #+END_SRC
GTS currently supports 1 kind of storage:
Example: #+BEGIN_SRC go storage := storage.NewPostgresStorage( storage.PostgresDBConfig{ DbURL: "postgresql://:@localhost:5432/?sslmode=disable", }, ) if err := storage.Connect(); err != nil { log.Fatal("Could not connect to db", err) }
if err := storage.Initialize(); err != nil { log.Fatal("Could not intialize database", err) } #+END_SRC
and then pass it to the scheduler.
Scheduling tasks can be done in 3 ways:
** Execute a task after 5 seconds. #+BEGIN_SRC go func MyFunc(arg1 string, arg2 string) taskID := s.RunAfter(5*time.Second, MyFunc, "Hello", "World") #+END_SRC
** Execute a task at a specific time. #+BEGIN_SRC go func MyFunc(arg1 string, arg2 string) taskID := s.RunAt(time.Now().Add(24 * time.Hour), MyFunc, "Hello", "World") #+END_SRC
** Execute a task every 1 minute. #+BEGIN_SRC go func MyFunc(arg1 string, arg2 string) taskID := s.RunEvery(1 * time.Minute, MyFunc, "Hello", "World") #+END_SRC
The [[https://github.com/ClubNFT/scheduler/tree/master/_example/][Examples]] folder contains a bunch of code samples you can look into.
GTS supports the ability to provide a custom storage, the newly created storage has to implement the TaskStore interface
#+BEGIN_SRC go type TaskStore interface { Store(task *TaskAttributes) error Remove(task *TaskAttributes) error Fetch() ([]TaskAttributes, error) } #+END_SRC
TaskAttributes looks as follows: #+BEGIN_SRC go type TaskAttributes struct { Hash string Name string LastRun string NextRun string Duration string IsRecurring string Params string } #+END_SRC
Credit This package is heavily inspired by [[https://github.com/agronholm/apscheduler/][APScheduler]] for Python & [[https://github.com/jasonlvhit/gocron][GoCron]]
License
MIT
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.