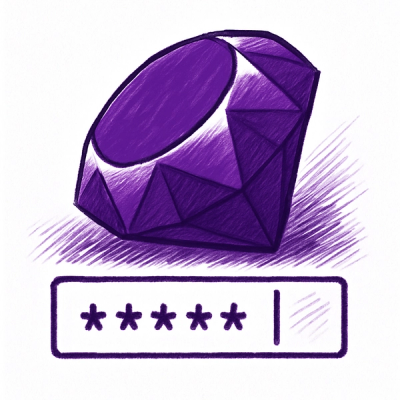
Research
/Security News
60 Malicious Ruby Gems Used in Targeted Credential Theft Campaign
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
github.com/codehack/go-strarr
Various operations for string slices for Go
Go-StrArr is a collection of functions that operate on string slices. Most are functions from package strings
that have been adapted to work with string slices, and some were ported from PHP array_* functions.
net/http
headers!Using "go get":
go get github.com/codehack/go-strarr
Then import:
import ("github.com/codehack/go-strarr")
package main
import(
"github.com/codehack/go-strarr"
"fmt"
)
// This example shows basic usage of various functions by manipulating
// the array 'arr'.
func main() {
arr := []string{"Go", "nuts", "for", "Go"}
foo := strarr.Repeat("Go",3)
fmt.Println(foo)
fmt.Println(strarr.Count(arr, "Go"))
fmt.Println(strarr.Index(arr, "Go"))
fmt.Println(strarr.LastIndex(arr, "Go"))
if strarr.Contains(arr, "nuts") {
arr = strarr.Replace(arr, []string{"Insanely"})
}
fmt.Println(arr)
str := strarr.Shift(&arr)
fmt.Println(str)
fmt.Println(arr)
strarr.Unshift(&arr, "Really")
fmt.Println(arr)
fmt.Println(strarr.ToUpper(arr))
fmt.Println(strarr.ToLower(arr))
fmt.Println(strarr.ToTitle(arr))
fmt.Println(strarr.Trim(arr,"Really"))
fmt.Println(strarr.Filter(arr,"Go"))
fmt.Println(strarr.Diff(arr,foo))
fmt.Println(strarr.Intersect(arr,foo))
fmt.Println(strarr.Rand(arr,2))
fmt.Println(strarr.Reverse(arr))
}
See the testing source strarr_test.go for more examples.
The full code documentation is located at GoDoc:
http://godoc.org/github.com/codehack/go-strarr
Go-StrArr is Copyright (c) 2014 Codehack. Published under MIT License
Go nuts for Go!
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
Security News
The CNA Scorecard ranks CVE issuers by data completeness, revealing major gaps in patch info and software identifiers across thousands of vulnerabilities.
Research
/Security News
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.