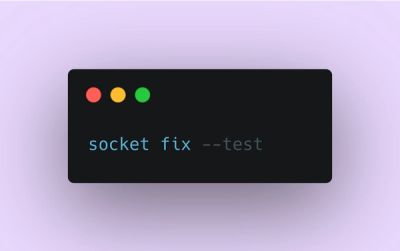
Product
Introducing Socket Fix for Safe, Automated Dependency Upgrades
Automatically fix and test dependency updates with socket fix—a new CLI tool that turns CVE alerts into safe, automated upgrades.
github.com/coming-chat/go-sui
Sui Golang SDK
The Sui Golang SDK for ComingChat. We welcome other developers to participate in the development and testing of sui-sdk.
go get github.com/coming-chat/go-sui
import "github.com/coming-chat/go-sui/account"
// Import account with mnemonic
acc, err := account.NewAccountWithMnemonic(mnemonic)
// Import account with private key
privateKey, err := hex.DecodeString("4ec5a9eefc0bb86027a6f3ba718793c813505acc25ed09447caf6a069accdd4b")
acc, err := account.NewAccount(privateKey)
// Get private key, public key, address
fmt.Printf("privateKey = %x\n", acc.PrivateKey[:32])
fmt.Printf(" publicKey = %x\n", acc.PublicKey)
fmt.Printf(" address = %v\n", acc.Address)
// Sign data
signedData := acc.Sign(data)
All data interactions on the Sui chain are implemented through the rpc client.
import "github.com/coming-chat/go-sui/client"
import "github.com/coming-chat/go-sui/types"
cli, err := client.Dial(rpcUrl)
// call JSON RPC
responseObject := uint64(0) // if response is a uint64
err := cli.CallContext(ctx, &responseObject, funcName, params...)
// e.g. call get transaction
digest, err := types.NewBase64Data("/KXvTwNRHKKzAB+/Dz1O64LjVbISgIW4VUCmuuPyEfU=")
resp := types.TransactionResponse{}
err := cli.CallContext(ctx, &resp, "sui_getTransaction", digest)
print("transaction status = ", resp.Effects.Status)
print("transaction timestamp = ", resp.TimestampMs)
// And you can call some predefined methods
digest, err := types.NewBase64Data("/KXvTwNRHKKzAB+/Dz1O64LjVbISgIW4VUCmuuPyEfU=")
resp, err := cli.GetTransaction(ctx, digest)
print("transaction status = ", resp.Effects.Status)
print("transaction timestamp = ", resp.TimestampMs)
We currently have some rpc methods built-in, see here
import "github.com/coming-chat/go-sui/client"
import "github.com/coming-chat/go-sui/types"
import "github.com/coming-chat/go-sui/account"
acc, err := account.NewAccountWithMnemonic(mnemonic)
signer, _ := types.NewAddressFromHex(acc.Address)
recipient, err := types.NewAddressFromHex("0x12345678.......")
suiObjectId, err := types.NewHexData("0x36d3176a796e167ffcbd823c94718e7db56b955f")
transferAmount := uint64(10000)
maxGasTransfer := 100
cli, err := client.Dial(rpcUrl)
txnBytes, err := cli.TransferSui(ctx, *signer, *recipient, suiObjectId, transferAmount, maxGasTransfer)
// Sign
signedTxn := txnBytes.SignWith(acc.PrivateKey)
txnResponse, err := cli.ExecuteTransaction(ctx, signedTxn)
print("transaction digest = ", txnResponse.Certificate.TransactionDigest)
print("transaction status = ", txnResponse.Effects.Status)
print("transaction gasFee = ", txnResponse.Effects.GasFee())
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Automatically fix and test dependency updates with socket fix—a new CLI tool that turns CVE alerts into safe, automated upgrades.
Security News
CISA denies CVE funding issues amid backlash over a new CVE foundation formed by board members, raising concerns about transparency and program governance.
Product
We’re excited to announce a powerful new capability in Socket: historical data and enhanced analytics.