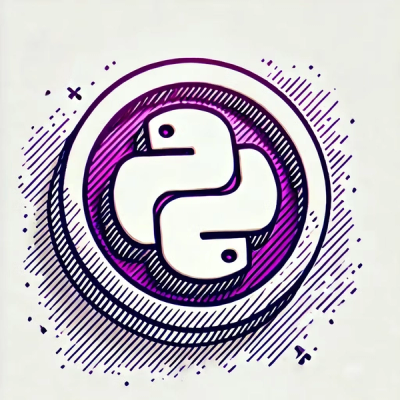
Product
Socket Now Supports pylock.toml Files
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
github.com/gladkikhartem/async
Go API & libraries for working with SLCT runtime, that allow Go developers to share asynchronous code (for example send message to Slack and return user input) with consistency and "only-once" processing guarantees.
package main
import (
"log"
"github.com/gladkikhartem/async"
"google.golang.org/grpc"
)
var defs = async.Definitions{
"counter": async.StandardDefinition(CounterProcess{}),
}
type CounterProcess struct {
Counter int64
}
func (c *CounterProcess) Main_Count(p *async.Process) error {
c.Counter++
p.Recv("counter").To(c.Main_Count).
.After(time.Hour).To(c.Main_Done)
return nil
}
func (c *CounterProcess) Main_Done(p *async.Process) error {
log.Print("done")
return nil
}
func main() {
conn, err := grpc.Dial("runtime_addr:9090", grpc.WithInsecure())
if err != nil {
log.Fatal(err)
}
defer conn.Close()
err := async.ManageDefs(context.Background(), conn, "1", defs)
if err != nil {
log.Fatal(err)
}
}
Asynchronous process is described as set of goroutines(FSM) with a shared state. In the code it's represented as Go struct with methods in a format {GoroutineName_FSMStatus}.
type ExampleProcess struct {
// local variables i.e. state
}
func (c *ExampleProcess) Main_Start(p *async.Process) error {
p.Go(c.Goroutine1_Start) // run parallel process in this state
return slack.Approve(p, c.Main_CheckApprove, "Approve?", time.Hour)
}
func (c *ExampleProcess) Main_CheckApprove(p *async.Process, result slack.Result) error {
// handle approval result
}
func (c *ExampleProcess) Goroutine1_Start(p *async.Process) error {
// ...
}
When async process is resumed:
Method can specify following unblocking conditions (same as in Go):
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.
Research
Security News
Malicious Ruby gems typosquat Fastlane plugins to steal Telegram bot tokens, messages, and files, exploiting demand after Vietnam’s Telegram ban.