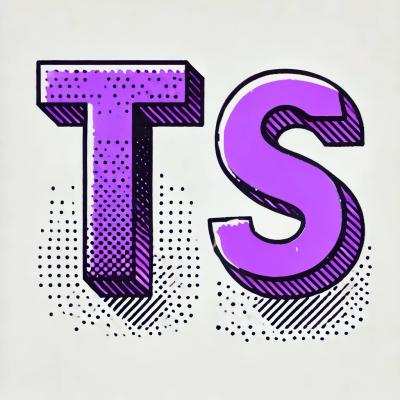
Security News
Node.js Moves Toward Stable TypeScript Support with Amaro 1.0
Amaro 1.0 lays the groundwork for stable TypeScript support in Node.js, bringing official .ts loading closer to reality.
github.com/joshburnsxyz/go-view-layouts
Supply Chain Security
Vulnerability
Quality
Maintenance
License
This Go library provides a thin wrapper around Go's html/template
package for managing and rendering HTML templates with layouts. It ensures thread-safe access to templates, allowing concurrent use in a web server environment.
go get github.com/joshburnsxyz/go-view-layouts
First, initialize the templates by providing a map of template names to file paths and the layout file path. This should be done once, typically at the start of your application.
package main
import (
"log"
"net/http"
layouts "github.com/joshburnsxyz/go-view-layouts"
)
func main() {
templateFiles := map[string]string{
"home": "templates/home.html",
"about": "templates/about.html",
"contact": "templates/contact.html",
}
err := layouts.Init(templateFiles, "templates/layout.html")
if err != nil {
log.Fatalf("Failed to initialize templates: %v", err)
}
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
layouts.RenderTemplate(w, "home", "layout", nil)
})
log.Fatal(http.ListenAndServe(":8080", nil))
}
To render a template, call the RenderTemplate
function with the response writer, template name, layout name, and data to be injected into the template.
package main
import (
"net/http"
layouts "github.com/joshburnsxyz/go-view-layouts"
)
func homeHandler(w http.ResponseWriter, r *http.Request) {
data := struct {
Title string
}{
Title: "Welcome Home",
}
layouts.RenderTemplate(w, "home", "layout", data)
}
func main() {
// Initialize templates
templateFiles := map[string]string{
"home": "templates/home.html",
"about": "templates/about.html",
"contact": "templates/contact.html",
}
err := layouts.Init(templateFiles, "templates/layout.html")
if err != nil {
log.Fatalf("Failed to initialize templates: %v", err)
}
// Setup handlers
http.HandleFunc("/", homeHandler)
http.HandleFunc("/about", func(w http.ResponseWriter, r *http.Request) {
layouts.RenderTemplate(w, "about", "layout", nil)
})
http.HandleFunc("/contact", func(w http.ResponseWriter, r *http.Request) {
layouts.RenderTemplate(w, "contact", "layout", nil)
})
// Start server
log.Fatal(http.ListenAndServe(":8080", nil))
}
If you find a bug or have a feature request, please open an issue on GitHub. Pull requests are welcome!
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Amaro 1.0 lays the groundwork for stable TypeScript support in Node.js, bringing official .ts loading closer to reality.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.