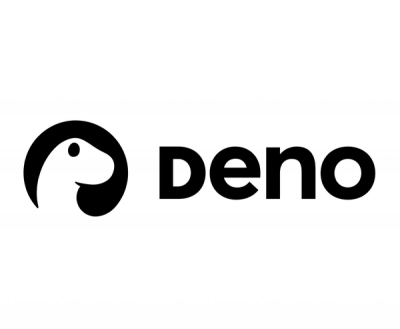
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
github.com/kwiscale/framework
Web Middleware for Golang
At this time, Kwiscale is at the very begining of developpement. But you can test and give'em some pull-request to improve it.
Install with "go get" command:
go get gopkg.in/kwiscale/framework.v1
Create a project:
mkdir ~/myproject && cd ~/myproject
The common way to create handlers is to append a package::
mkdir handlers
vim handlers/index.go
Let's try an example:
package handlers
import "gopkg.in/kwiscale/framework.v1"
// this is the Index Handler that
// is composed by a RequestHandler
type IndexHandler struct {
// compose your handler with kwiscale.Handler
kwiscale.RequestHandler
}
// Will respond to GET request. "params" are url params (not GET and POST data)
func (i *IndexHandler) Get () {
i.WriteString("Hello !" + i.Vars["userid"])
}
Then in you main.go::
package main
import (
"gopkg.in/kwiscale/framework.v1"
"./handlers"
)
// HomeHandler
type HomeHandler struct {
kwiscale.RequestHandler
}
// Get respond to GET request
func (h *HomeHandler) Get (){
h.WriteString("reponse to GET home")
}
// Another handler
type OtherHandler struct {
kwiscale.RequestHandler
}
func (o *OtherHandler) Get (){
// read url params
// it always returns a string !
userid := o.Vars["userid"]
o.WriteString(fmt.Printf("Hello user %s", userid))
}
func main() {
kwiscale.DEBUG = true
app := kwiscale.NewApp(&kswicale.Config{
Port: ":8000",
})
app.AddRoute("/", HomeHandler{})
app.AddRoute("/user/{userid:[0-9]+}", OtherHandler{})
app.ListenAndServe()
// note: App respects http.Mux so you can use:
// http.ListenAndServe(":9999", app)
// to override behaviors, testing, or if your infrastructure
// restricts this usage
}
Then run:
go run main.go
Or build your project:
go build main.go
./main
Kwiscale let you declare Handler methods with the HTTP method. This allows you to declare:
Kwiscale provides a "basic" template engine that use http/template
. Kwiscale only add a "very basic template override system".
If you plan to have a complete override system, please use http://gopkg.in/kwiscale/template-pongo2.v1 that implements pango2 template.
See the following example.
Append templates directory:
mkdir templates
Then create templates/main.html:
<!DOCTYPE html>
<html>
<head>
<title>{{ if .title }}{{.title}}{{ else }} Default title {{ end }}</title>
</head>
<body>
{{/* Remember to use "." as context */}}
{{ template "CONTENT" . }}
</body>
</html>
Now create templates/home directory:
mkdir templates/home
Create templates/home/welcome.html:
{{/* override "main.html" */}}
{{ define "CONTENT" }}
This the welcome message {{ .msg }}
{{ end }}
This template overrides "main.html" (in ./templates/
directory) and append "CONTENT" template definition. So, the "CONTENT" block will appear at template "CONTENT"
in "main.html". That's all.
In handlers/index.go you may now ask for template rendering:
func (h *IndexHandler) Get() {
h.Render("home/welcome.html", map[string]string{
"title" : "Welcome !!!",
"msg" : "Hello you",
})
}
You can override template directory using App configuration passed to the constructor:
app := kwiscale.NewApp(&kswiscale.Config{
TemplateDir: "./my-template-dir",
})
Features in progress:
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.