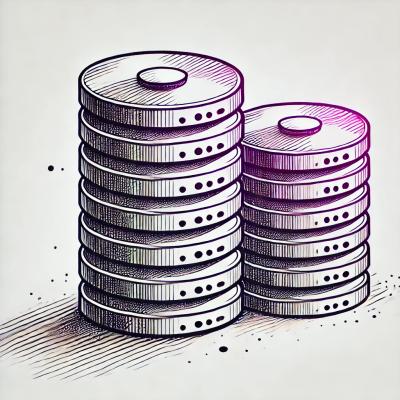
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
github.com/lafriks/go-tiled
Go library to parse Tiled map editor file format (TMX) and render map to image. Currently supports only orthogonal finite maps rendering out-of-the-box.
go get github.com/lafriks/go-tiled
You can use go get -u
to update the package. You can also just import and start using the package directly if you're using Go modules, and Go will then download the package on first compilation.
package main
import (
"fmt"
"os"
"github.com/lafriks/go-tiled"
"github.com/lafriks/go-tiled/render"
)
const mapPath = "maps/map.tmx" // Path to your Tiled Map.
func main() {
// Parse .tmx file.
gameMap, err := tiled.LoadFile(mapPath)
if err != nil {
fmt.Printf("error parsing map: %s", err.Error())
os.Exit(2)
}
fmt.Println(gameMap)
// You can also render the map to an in-memory image for direct
// use with the default Renderer, or by making your own.
renderer, err := render.NewRenderer(gameMap)
if err != nil {
fmt.Printf("map unsupported for rendering: %s", err.Error())
os.Exit(2)
}
// Render just layer 0 to the Renderer.
err = renderer.RenderLayer(0)
if err != nil {
fmt.Printf("layer unsupported for rendering: %s", err.Error())
os.Exit(2)
}
// Get a reference to the Renderer's output, an image.NRGBA struct.
img := renderer.Result
// Clear the render result after copying the output if separation of
// layers is desired.
renderer.Clear()
// And so on. You can also export the image to a file by using the
// Renderer's Save functions.
}
For further documentation, see https://pkg.go.dev/github.com/lafriks/go-tiled or run:
godoc github.com/lafriks/go-tiled
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.