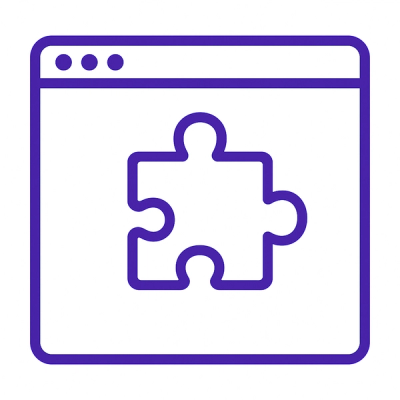
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
github.com/laik/timerqueue
Supply Chain Security
Vulnerability
Quality
Maintenance
License
The timerqueue package implements a priority queue for objects scheduled to perform actions at clock times.
See http://godoc.org/github.com/beevik/timerqueue for godoc-formatted API documentation.
The following code declares an object implementing the Timer interface, creates a timerqueue, and adds three events to the timerqueue.
type event int
func (e event) OnTimer(t time.Time) {
fmt.Printf("event.OnTimer %d fired at %v\n", int(e), t)
}
queue := timerqueue.New()
queue.Schedule(event(1), time.Date(2015, 1, 1, 0, 0, 0, 0, time.UTC))
queue.Schedule(event(2), time.Date(2015, 1, 3, 0, 0, 0, 0, time.UTC))
queue.Schedule(event(3), time.Date(2015, 1, 2, 0, 0, 0, 0, time.UTC))
Using the queue initialized in the first example, the following code examines the head of the timerqueue and outputs the id and time of the event found there.
e, t := queue.PeekFirst()
if e != nil {
fmt.Printf("Event %d will be first to fire at %v.\n", int(e.(event)), t)
fmt.Printf("%d events remain in the timerqueue.", queue.Len())
}
Output:
Event 1 will be first to fire at 2015-01-01 00:00:00 +0000 UTC.
3 events remain in the timerqueue.
Using the queue initialized in the first example, this code removes the next timer to be executed until the queue is empty.
for queue.Len() > 0 {
e, t := queue.PopFirst()
fmt.Printf("Event %d fires at %v.\n", int(e.(event)), t)
}
Output:
Event 1 fires at 2015-01-01 00:00:00 +0000 UTC.
Event 3 fires at 2015-01-02 00:00:00 +0000 UTC.
Event 2 fires at 2015-01-03 00:00:00 +0000 UTC.
The final example shows how to dispatch OnTimer callbacks to timers using the timerqueue's Advance method.
Advance calls the OnTimer method for each timer scheduled before the requested time. Timers are removed from the timerqueue in order of their scheduling.
// Call the OnTimer method for each event scheduled before
// January 10, 2015. Pop the called timer from the queue.
queue.Advance(time.Date(2015, 1, 10, 0, 0, 0, 0, time.UTC))
Output:
event.OnTimer 1 fired at 2015-01-01 00:00:00 +0000 UTC.
event.OnTimer 3 fired at 2015-01-02 00:00:00 +0000 UTC.
event.OnTimer 2 fired at 2015-01-03 00:00:00 +0000 UTC.
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.