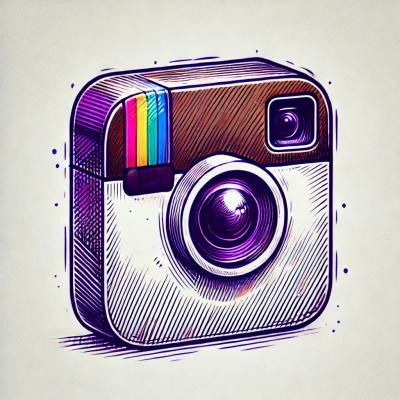
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
github.com/lattots/nlp
NLP is a natural language processing library for Go. It is designed to make text processing easier for machine learning use cases.
TODO: Write installation instructions
package main
import (
"fmt"
"log"
"github.com/lattots/nlp/pkg/tokenizer"
)
func main() {
language := "eng" // Target language for tokenizer
tok, err := tokenizer.New(language)
if err != nil {
log.Fatalln("error creating tokenizer:", err)
}
exampleText := "This is some example text. It is used to showcase package tokenizer."
tokens := tok.GetTokens(exampleText)
fmt.Println(tokens)
lemmas, err := tok.Lemmatize(tokens)
if err != nil {
log.Fatalln("error lemmatizing tokens:", err)
}
fmt.Println(lemmas)
sentences := tok.GetSentences(exampleText)
fmt.Println(sentences)
}
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.