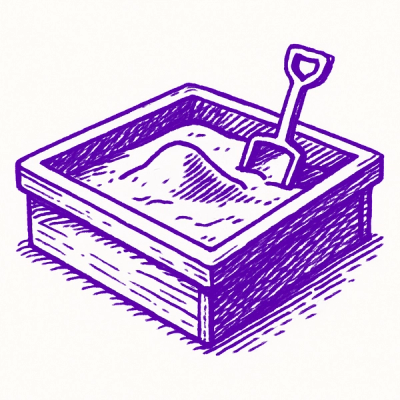
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
github.com/markoxley/mud
Note: This is a work in progress and is not yet ready for production use.
mud is a versatile and lightweight ORM (Object-Relational Mapping) package for Go that supports multiple database backends including PostgreSQL, MySQL, SQLite, and Microsoft SQL Server.
go get github.com/markoxley/mud
package main
import (
"github.com/markoxley/mud"
"github.com/markoxley/mud/where"
)
// Define your model
type User struct {
mud.Model
Username string `mud:"size:64,key:true"`
Email string `mud:"size:256"`
}
func main() {
// Initialize database connection
config := mud.Config{
Driver: "mysql",
Host: "localhost",
Port: 3306,
Database: "mydb",
Username: "user",
Password: "password",
}
db, err := mud.Connect(config)
if err != nil {
panic(err)
}
defer db.Close()
// Create a new user
user := &User{
Username: "johndoe",
Email: "john@example.com",
}
err = db.Save(user)
if err != nil {
panic(err)
}
// Query users with WHERE clause
var users []User
where := where.Equal("username", "johndoe")
err = db.Find(&users, where)
if err != nil {
panic(err)
}
// Update user, we reuse the Save method
user.Email = "john.doe@example.com"
err = db.Save(user)
if err != nil {
panic(err)
}
// Delete user
err = db.Remove(user)
if err != nil {
panic(err)
}
// Convenience functions with generics
user, err = mud.First[User](db, where.Equal("username", "johndoe"))
if err != nil {
panic(err)
}
user, err = mud.FromID[User](db, *user.ID)
if err != nil {
panic(err)
}
}
mud provides a powerful WHERE clause builder with support for various conditions:
// Basic conditions
where.Equal("field", value)
where.NotEqual("field", value)
where.Greater("field", value)
where.Less("field", value)
// String operations
where.Contains("field", "substring")
where.StartsWith("field", "prefix")
where.EndsWith("field", "suffix")
// Null checks
where.IsNull("field")
where.NotIsNull("field")
// Range operations
where.Between("field", value1, value2)
where.In("field", []interface{}{value1, value2})
// Combining conditions
where.Equal("field1", value1).AndEqual("field2", value2)
where.Equal("field1", value1).OrEqual("field2", value2)
mud provides a powerful ORDER BY clause builder with support for various conditions:
// Basic conditions
order.Asc("field")
order.Desc("field")
// Combining conditions
order.Asc("field1").Desc("field2")
mud uses struct tags to define model properties:
mud:""
- Specify the field is to be included in the databasemud:"key:true"
- Create an index on fieldmud:"size:255"
- Set field sizemud:"allowNull"
- Allow NULL valuesThis project is licensed under the MIT License - see the LICENSE file for details.
Contributions are welcome! Please feel free to submit a Pull Request.
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.