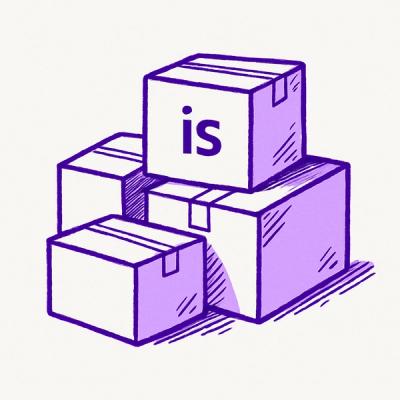
Security News
npm ‘is’ Package Hijacked in Expanding Supply Chain Attack
The ongoing npm phishing campaign escalates as attackers hijack the popular 'is' package, embedding malware in multiple versions.
github.com/mccutchen/go-httpbin
A reasonably complete and well-tested golang port of Kenneth Reitz's httpbin service, with zero dependencies outside the go stdlib.
Run as a standalone binary, configured by command line flags or environment variables:
$ go-httpbin -help
Usage of ./dist/go-httpbin:
-max-duration duration
Maximum duration a response may take (default 10s)
-max-memory int
Maximum size of request or response, in bytes (default 1048576)
-port int
Port to listen on (default 8080)
Docker images are published to Docker Hub:
$ docker run -P mccutchen/go-httpbin
The github.com/mccutchen/go-httpbin/httpbin
package can also be used as a
library for testing an applications interactions with an upstream HTTP service,
like so:
package httpbin_test
import (
"net/http"
"net/http/httptest"
"testing"
"time"
"github.com/mccutchen/go-httpbin/httpbin"
)
func TestSlowResponse(t *testing.T) {
handler := httpbin.NewHTTPBin().Handler()
srv := httptest.NewServer(handler)
defer srv.Close()
client := http.Client{
Timeout: time.Duration(1 * time.Second),
}
_, err := client.Get(srv.URL + "/delay/10")
if err == nil {
t.Fatal("expected timeout error")
}
}
go get github.com/mccutchen/go-httpbin/...
I've been a longtime user of Kenneith Reitz's original httpbin.org, and wanted to write a golang port for fun and to see how far I could get using only the stdlib.
When I started this project, there were a handful of existing and incomplete
golang ports, with the most promising being ahmetb/go-httpbin. This
project showed me how useful it might be to have an httpbin
library
available for testing golang applications.
Compared to the original:
/brotli
endpoint (due to lack of support in Go's stdlib)?show_env=1
query param is ignored (i.e. no special handling of
runtime environment headers)Compared to ahmetb/go-httpbin:
# local development
make
make test
make testcover
make run
# building & pushing docker images
make image
make imagepush
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The ongoing npm phishing campaign escalates as attackers hijack the popular 'is' package, embedding malware in multiple versions.
Security News
A critical flaw in the popular npm form-data package could allow HTTP parameter pollution, affecting millions of projects until patched versions are adopted.
Security News
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.