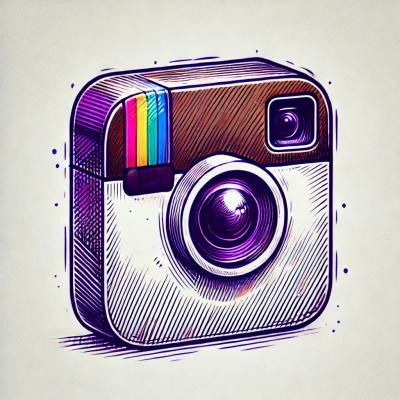
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
github.com/mikaelim-id/go-query-string-builder
go-query-string-builder is Go library for building query string
import "github.com/mikaelim-id/go-query-string-builder/query"
selectQuery := SelectQuery{
SelectStatement: []string{"*",},
FromCommand: "test_table",
WhereClause: "name='adama",
GroupByClause: []string{"name"},
OrderByClause: []OrderBy{
{
Field: "name",
Asc: false,
},
{
Field: "address",
Asc: true,
},
},
Limit: 5,
Offset: nil,
}
Append Field = Value and condition in SelectQuery, will be ignored if value is empty.
selectQuery.AppendAndEqualCondition("name","name_value")
Append and condition in SelectQuery.
selectQuery.AppendAndCondition("name=name_value")
Append Field = Value or condition in SelectQuery, will be ignored if value is empty.
selectQuery.AppendOrEqualCondition("name","name_value")
Append or condition in SelectQuery.
selectQuery.AppendOrCondition("name=name_value")
selectQuery.build()
pointer := 1
offset := 1
selectQuery := query.SelectQuery{
SelectStatement: []string{"name", "address", "phone", "date_of_birth", "location_id"},
FromCommand: "test_table",
GroupByClause: []string{"name", "address"},
OrderByClause: []OrderBy{
{
Field: "name",
Asc: false,
},
{
Field: "address",
Asc: true,
},
},
Limit: 5,
Offset: &offset,
}
selectQuery.AppendAndEqualCondition("name", "test_name")
selectQuery.AppendAndEqualCondition("value", 1)
selectQuery.AppendAndEqualCondition("pointer", &pointer)
selectQuery.AppendAndEqualCondition("address", nil)
selectQuery.AppendAndCondition("phone is not null")
selectQuery.AppendOrCondition(query.BuildGroupedOrCondition("location_id=1", "location_id=3"))
fmt.Println(selectQuery.build())
// select name,address,phone,date_of_birth,location_id from test_table where
// name='test_name' and value=1 and pointer='1' and phone is not null or (location_id=1 or location_id=3)
// group by name,address order by name desc,address asc limit 5 offset 1
updateQuery := UpdateQuery{
UpdateStatement: "test_table",
SetCommand: map[string]string{
"name": "new name value",
},
WhereClause: "name='adam'",
}
Append Field = Value set in UpdateQuery, will be ignored if value is empty.
updateQuery.AppendSet("address","new avenue")
Append Field = Value set in UpdateQuery.
updateQuery.AppendSet("address","new avenue")
Append Field = Value and condition in SelectQuery, will be ignored if value is empty.
selectQuery.AppendAndEqualCondition("name","name_value")
Append and condition in SelectQuery.
selectQuery.AppendAndCondition("name=name_value")
Append Field = Value or condition in SelectQuery, will be ignored if value is empty.
selectQuery.AppendOrEqualCondition("name","name_value")
Append or condition in SelectQuery.
selectQuery.AppendOrCondition("name=name_value")
updateQuery.build()
jsonbSetPtr := `jsonb_set(additional_data,'{review_by}','"test"')`
updateQuery := query.UpdateQuery{
UpdateStatement: "test_table",
}
updateQuery.AppendSet("name", "nama")
updateQuery.AppendSet("value", "val")
updateQuery.AppendSet("location_id", "loc")
updateQuery.AppendSet("form_data", `jsonb_set(form_data,'{additional_data,review_by}','"test"')`)
updateQuery.AppendSet("client_data", &jsonbSetPtr)
updateQuery.AppendAndEqualCondition("name", "test")
updateQuery.AppendAndEqualCondition("location_id", "test")
updateQuery.AppendAndEqualCondition("value", 1)
fmt.Println(updateQuery.build())
// update test_table set name=:name,value=:value,location_id=:location_id,
// form_data=jsonb_set(form_data,'{additional_data,review_by}','"test"'),
// client_data=jsonb_set(additional_data,'{review_by}','"test"')
// where name='test' and location_id='test' and value=1
Create grouped and condition.
groupeAndCondition := selectQuery.BuildGroupedAndCondition("name='adam'", "name='eve'")
fmt.Println(groupedAndCondition)
// (name='adam' and name='eve')
Create grouped or condition.
groupedOrCondition := selectQuery.BuildGroupedOrCondition("name='adam'", "name='eve'")
fmt.Println(groupedOrCondition)
// (name='adam' or name='eve')
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.