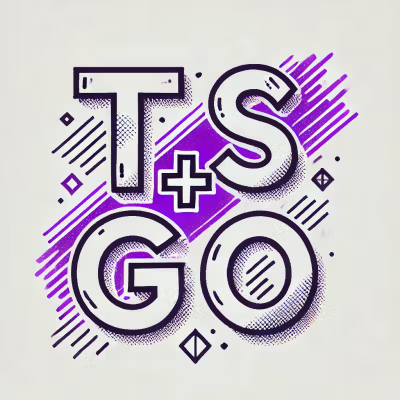
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
github.com/monkhai/telelogger-golang
A lightweight, easy-to-use Telegram logging utility for Go applications.
go get github.com/monkhai/telelogger-golang
package main
import "github.com/monkhai/telelogger-golang"
func main() {
logger := telelogger.New(telelogger.Config{
BotToken: "YOUR_BOT_TOKEN",
ChatID: YOUR_CHAT_ID,
})
// Basic logging
logger.LogInfo("Hello, world!")
logger.LogError("Something went wrong!")
logger.LogSuccess("Operation completed successfully!")
logger.LogWarn("Warning: Resource running low")
}
The New
function accepts a Config
struct with the following options:
type Config struct {
// Your Telegram Bot Token
BotToken string
// Target Chat ID where messages will be sent
ChatID int64
// The formatting of the message
// Can be ParseModeHTML, ParseModeMarkdown, or ParseModeMarkdownV2
ParseMode ParseMode
// Custom formatter for info messages
InfoFormatter FormatterFunc
// Custom formatter for error messages
ErrorFormatter FormatterFunc
// Custom formatter for success messages
SuccessFormatter FormatterFunc
// Custom formatter for warning messages
WarnFormatter FormatterFunc
}
// FormatterFunc is a function type for message formatting
type FormatterFunc func(message string) string
You can customize how messages are formatted before they're sent to Telegram.
Notice the <b>
tags in the formatters, adding bold text to the titles.
This allows you to add more information to the messages, such as links, bold text, etc.
For more information on the different parse modes, see the Telegram API documentation.
logger := telelogger.New(telelogger.Config{
BotToken: "YOUR_BOT_TOKEN",
ChatID: YOUR_CHAT_ID,
ParseMode: telelogger.ParseModeHTML, // allows us to add <b> tags and more
InfoFormatter: func(msg string) string {
return fmt.Sprintf("ℹ️ <b>INFO:</b>\n%s", msg)
},
ErrorFormatter: func(msg string) string {
return fmt.Sprintf("❌ <b>ERROR:</b>\n%s", msg)
},
SuccessFormatter: func(msg string) string {
return fmt.Sprintf("✅ <b>SUCCESS:</b>\n%s", msg)
},
WarnFormatter: func(msg string) string {
return fmt.Sprintf("🚨️ <b>WARNING:</b>\n%s", msg)
},
})
Unlike the TypeScript version, this package follows Go's error handling patterns:
// All logging methods return an error that you can handle
if err := logger.LogInfo("Hello, world!"); err != nil {
// Handle error
}
// You can pass either a string or an error to LogError
err := someFunction()
if err != nil {
logger.LogError(err) // Accepts error interface
}
logger.LogError("Something went wrong") // Also accepts string
MIT
Contributions are welcome! Please feel free to submit a Pull Request.
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.