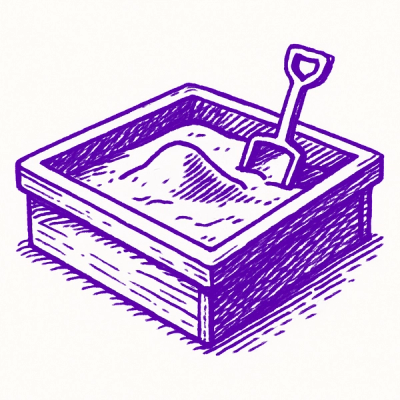
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
github.com/nanoninja/render
A flexible Go package for rendering content in different formats with configurable options and formatting.
go get github.com/nanoninja/render
// Create renderer
renderer := render.JSON()
data := map[string]string{"message": "ping"}
// Simple render
renderer.Render(os.Stdout, data)
// Pretty printed with custom indent
renderer.Render(os.Stdout, data, render.Format(
render.Pretty(),
render.Indent(" "),
))
// Simple text
render.Text().Render(os.Stdout, "Hello World")
// With formatting
render.Text().Render(os.Stdout, "Hello %s", render.Textf("Gopher"))
// Create buffered JSON renderer
render.Buffer(render.JSON()).Render(os.Stdout, data)
package main
import (
"embed"
"net/http"
"strings"
"text/template"
"github.com/nanoninja/render"
"github.com/nanoninja/render/tmpl"
"github.com/nanoninja/render/tmpl/loader"
)
//go:embed templates
var templatesFS embed.FS
func main() {
// Create a loader for your templates
src := loader.NewEmbed(templatesFS, tmpl.LoaderConfig{
Root: "templates",
Extension: ".html",
})
// Create template with configuration
t := tmpl.HTML("",
tmpl.SetFuncsHTML(template.FuncMap{
"upper": strings.ToUpper,
}),
tmpl.LoadHTML(src),
)
// Use in an HTTP handler
http.HandleFunc("/", func(w http.ResponseWriter, r *http.Request) {
data := map[string]any{"Title": "Welcome"}
t.RenderContext(r.Context(), w, data,
render.Name("index.html"), // Specify which template to render
render.WriteResponse(w), // Write headers to response
)
})
http.ListenAndServe("localhost:8080", nil)
}
ctx, cancel := context.WithTimeout(context.Background(), 5*time.Second)
defer cancel()
renderer.RenderContext(ctx, w, data)
Options can be configured using functional options:
// Pretty printing with custom format
renderer.Render(w, data, render.Format(
render.Pretty(), // Enable pretty printing
render.Indent(" "), // Custom indentation
render.LineEnding("\n"), // Line ending style
render.Prefix("// ") // Line prefix
))
// CSV specific options
renderer.Render(w, data, render.Format(
render.Separator(";"), // Use semicolon as separator
render.UseCRLF(), // Use Windows-style line endings
))
// Set template name (for template renderers)
renderer.Render(w, data, render.Name("index.html"))
// Set render timeout
renderer.Render(w, data, render.Timeout(5*time.Second))
// Custom parameters
renderer.Render(w, data, render.Param("key", "value"))
// Combining multiple options
renderer.Render(w, data, render.With(
render.MimeJSON(),
render.Format(render.Pretty()),
render.Timeout(5*time.Second),
))
func handleJSON(w http.ResponseWriter, r *http.Request) {
data := struct {
Message string `json:"message"`
}{
Message: "Hello, World!",
}
renderer := render.JSON()
renderer.Render(w, data,
render.MimeJSON(), // Set Content-Type
render.WriteResponse(w), // Write headers to response
render.Format(render.Pretty()) // Pretty print output
)
}
You can create your own renderers to support any output format. Here's a complete guide to implementing a custom renderer.
Your renderer must implement the Renderer
interface. Here's a minimal example:
type CustomRenderer struct {}
func (r *CustomRenderer) Render(w io.Writer, data any, opts func(*render.Options)) error {
return r.RenderContext(context.Background(), w, data, opts...)
}
func (r *CustomRenderer) RenderContext(ctx context.Context, w io.Writer, data any, opts ...func(*render.Options)) error {
// Check context validity
if err := render.CheckContext(ctx); err != nil {
return err
}
// Get options with default values
opt := render.NewOptions(opts...)
// Your rendering logic here
return nil
}
NewOptions
: Processes option functions and returns configured OptionsCheckContext
: Verifies if the context is still valid
Common option handlers for content type, formatting, etc.This project is licensed under the BSD 3-Clause License.
It allows you to:
The only requirements are:
For more details, see the LICENSE file in the project repository.
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.