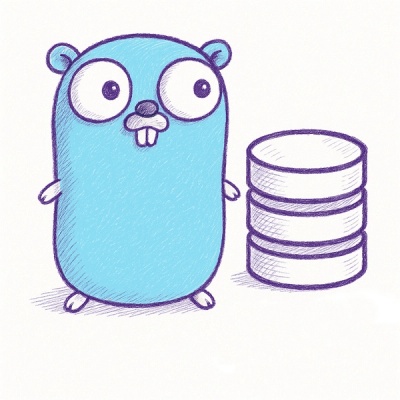
Security News
Official Go SDK for MCP in Development, Stable Release Expected in August
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
github.com/popgogo/sql-query-builder
The QueryBuilder
package provides a flexible and intuitive way to construct SQL queries programmatically in Go. It is designed to simplify the process of building complex queries by offering a chainable API for defining query components such as fields, conditions, joins, and Common Table Expressions (CTEs).
To start building a query, create a new QueryBuilder
instance by specifying the table name:
qb := querybuilder.NewQueryBuilder("users")
Specify the fields to include in the SELECT statement:
qb.Select("id", "name", "email")
Add conditions to the WHERE clause using Where
and OrWhere
methods:
qb.Where("age", ">", 18).OrWhere("status", "=", "active")
Define relationships between tables using the Join
method:
qb.Join("orders", "id", "user_id")
Include CTEs in your query using the AddCTE
method:
cte := querybuilder.NewQueryBuilder("recent_orders").Select("id", "user_id").Where("created_at", ">", "2025-01-01")
qb.AddCTE("recent_orders_cte", cte)
Generate the final SQL query and its arguments:
query, args := qb.BuildQuery()
fmt.Println("Query:", query)
fmt.Println("Arguments:", args)
Here is a complete example of building a query:
qb := querybuilder.NewQueryBuilder("users")
qb.Select("users.id", "users.name", "orders.total")
.Join("orders", "id", "user_id")
.Where("users.age", ">", 18)
.OrWhere("users.status", "=", "active")
query, args := qb.BuildQuery()
fmt.Println("Query:", query)
fmt.Println("Arguments:", args)
NewQueryBuilder(tableName string) *QueryBuilder
Creates a new QueryBuilder
instance for the specified table.
Select(fields ...string) *QueryBuilder
Specifies the fields to include in the SELECT statement.
Where(field, operator string, value interface{}) *QueryBuilder
Adds a condition to the WHERE clause.
OrWhere(field, operator string, value interface{}) *QueryBuilder
Adds an OR condition to the WHERE clause.
Join(table, foreignKey, primaryKey string) *QueryBuilder
Adds a JOIN clause to the query.
AddCTE(name string, queryBuilder *QueryBuilder) *QueryBuilder
Adds a Common Table Expression (CTE) to the query.
BuildQuery() (string, []interface{})
Generates the final SQL query and its arguments.
This package is open-source and available under the MIT License.
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.