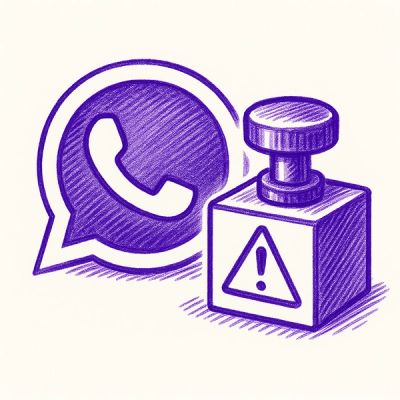
Research
/Security News
Malicious npm Packages Target WhatsApp Developers with Remote Kill Switch
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
github.com/shubhamsnehi/convert-json-api-to-csv-golang
Here you will find a easy program to convert a JSON response API to a CSV File
No need to install any package explictly. Just copy paste the follwoing import in main() of main.go.
import (
"encoding/csv"
"encoding/json"
"fmt"
"io/ioutil"
"log"
"net/http"
"os"
"time"
)
//Api URL
url := "http://localhost:3000/orderdata"
spaceClient := http.Client{
Timeout: time.Second * 2, // Timeout after 2 seconds
}
//Api GET call
req, err := http.NewRequest(http.MethodGet, url, nil)
if err != nil {
log.Fatal(err)
}
req.Header.Set("User-Agent", "spacecount-tutorial")
res, getErr := spaceClient.Do(req)
if getErr != nil {
log.Fatal(getErr)
}
if res.Body != nil {
defer res.Body.Close()
}
body, readErr := ioutil.ReadAll(res.Body)
if readErr != nil {
log.Fatal(readErr)
}
Order := Orders{}
//Un Marshalling JSON response content to 'Order'
jsonErr := json.Unmarshal(body, &Order)
if jsonErr != nil {
log.Fatal(jsonErr)
}
fmt.Println("Successfully read JSON API")
// Open our jsonFile
jsonFile, err := os.Open("OrderDetails.json")
// Handle error then handle it
if err != nil {
fmt.Println(err)
}
fmt.Println("Successfully Opened OrderDetails.json")
// defer the closing of our jsonFile so that we can parse it later on
defer jsonFile.Close()
// read our opened jsonFile as a byte array.
byteValue, _ := ioutil.ReadAll(jsonFile)
//initialize struct
var Order Orders
// jsonFile's content into 'Order' which we defined above
err = json.Unmarshal(byteValue, &Order)
if err != nil {
fmt.Println(err)
}
//Create CSV File
csvFile, err := os.Create("./OrderDetails.csv")
if err != nil {
fmt.Println(err)
}
defer csvFile.Close()
writer := csv.NewWriter(csvFile)
var row []string
row = append(row, Order.OrdersummaryBySupplierid.Supplier.City)
row = append(row, Order.OrdersummaryBySupplierid.Supplier.Name)
row = append(row, Order.OrdersummaryBySupplierid.Supplier.State)
writer.Write(row)
//Appending nested struct
for _, emp := range Order.OrdersummaryBySupplierid.Orderstatus {
var row1 []string
row1 = append(row1, emp.Orderdate)
row1 = append(row1, emp.Billed)
row1 = append(row1, emp.Bounced)
row1 = append(row1, emp.Pending)
writer.Write(row1)
}
// remember to flush!
writer.Flush()
You can change the delimeter '|' of .csv format by ctrl + Click on writer.Write() function
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
Research
/Security News
Socket uncovered 11 malicious Go packages using obfuscated loaders to fetch and execute second-stage payloads via C2 domains.
Security News
TC39 advances 11 JavaScript proposals, with two moving to Stage 4, bringing better math, binary APIs, and more features one step closer to the ECMAScript spec.