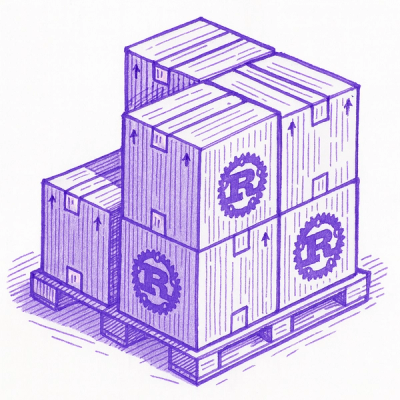
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
github.com/singchia/go-timer/v2
A high performance timer with minimal goroutines.
package main
import (
"log"
"time"
timer "github.com/singchia/go-timer/v2"
)
func main() {
t1 := time.Now()
// new timer
t := timer.NewTimer()
// add a tick in 1s
tick := t.Add(time.Second)
// wait for it
<-tick.C()
// tick fired as time is up, calculate and print the elapse
log.Printf("time elapsed: %fs\n", time.Now().Sub(t1).Seconds())
}
package main
import (
"log"
"sync"
"time"
timer "github.com/singchia/go-timer/v2"
)
func main() {
// we need a wait group since using async handler
wg := sync.WaitGroup{}
wg.Add(1)
// new timer
t := timer.NewTimer()
// add a tick in 1s with current time and a async handler
t.Add(time.Second, timer.WithData(time.Now()), timer.WithHandler(func(event *timer.Event) {
defer wg.Done()
// tick fired as time is up, calculate and print the elapse
log.Printf("time elapsed: %fs\n", time.Now().Sub(event.Data.(time.Time)).Seconds())
}))
wg.Wait()
}
package main
import (
"log"
"time"
timer "github.com/singchia/go-timer/v2"
)
func main() {
t1 := time.Now()
// new timer
t := timer.NewTimer()
// add cyclical tick in 1s
tick := t.Add(time.Second, timer.WithCyclically())
for {
// wait for it cyclically
<-tick.C()
t2 := time.Now()
// calculate and print the elapse
log.Printf("time elapsed: %fs\n", t2.Sub(t1).Seconds())
t1 = t2
}
}
© Austin Zhai, 2015-2025
Released under the Apache License 2.0
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.