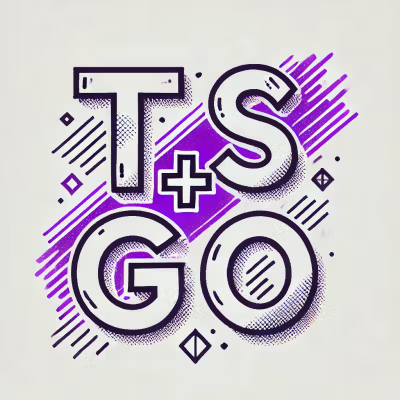
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
github.com/tris/weatherflow
weatherflow is a Go module for streaming rapid observations from the WeatherFlow Tempest API.
go get -u github.com/tris/weatherflow
import (
"fmt"
"log"
"github.com/tris/weatherflow"
)
func main() {
client := weatherflow.NewClient("your-token-here", nil, log.Printf)
client.AddDevice(12345)
client.Start(func(msg weatherflow.Message) {
switch m := msg.(type) {
case *weatherflow.MessageObsSt:
fmt.Printf("Observation: %+v\n", m)
case *weatherflow.MessageRapidWind:
fmt.Printf("Rapid wind: %+v\n", m)
}
})
time.Sleep(30 * time.Second)
client.Stop()
}
obs_st
in a batch which are up to 10 minutes old)I took a bit of inspiration from the excellent goweatherflow module, which you should use instead if your Tempest is on the same LAN.
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.