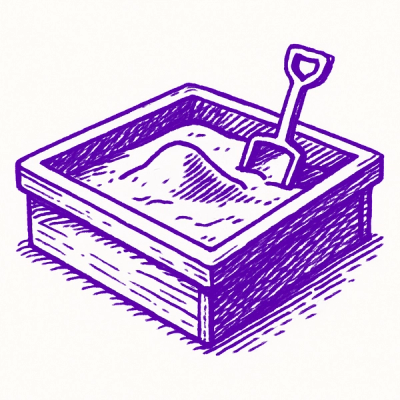
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
github.com/ujjvalrajput/post-quantum-cryptography-experimentation
This project demonstrates how to integrate AES encryption and RSA digital signatures into a Go program. The example ensures the message is protected and verified, making it suitable for Crypto Agile applications.
Clone the repository or download the main.go
file to your local machine.
Navigate to the directory where the main.go
file is located.
cd path/to/your/directory
Set the AES_KEY
environment variable. This key will be used for AES encryption. Replace thisis16byteskey
with your desired key.
On Linux or macOS:
export AES_KEY=thisis16byteskey
On Windows (Command Prompt):
set AES_KEY=thisis16byteskey
On Windows (PowerShell):
$env:AES_KEY="thisis16byteskey"
To run the program, use the go run
command:
go run main.go
export AES_KEY=thisis16byteskey
go run main.go
set AES_KEY=thisis16byteskey
go run main.go
$env:AES_KEY="thisis16byteskey"
go run main.go
If everything is set up correctly, you should see output similar to the following:
Original Message: Hello, Crypto Agile!
Encrypted Message: [hex-encoded encrypted message]
SHA-256 Hash: [calculated hash]
RSA Signature: [hex-encoded RSA signature]
Signature Verification: true
encryptAES
function encrypts a plaintext message using AES encryption.pad
function pads the plaintext to ensure it is a multiple of the AES block size. The unpad
function removes this padding.calculateHash
function calculates the SHA-256 hash of the input.generateRSAKeyPair
function generates an RSA key pair.signRSA
function signs the hashed message using the RSA private key.verifyRSA
function verifies the RSA signature using the RSA public key.FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.