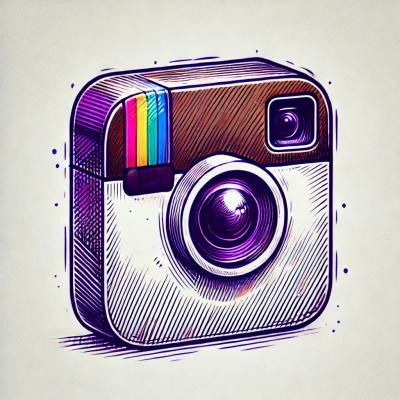
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
github.com/zhangzqs/gorm-async-task
基于GORM的分布式异步任务调度框架,提供可靠的状态管理和弹性任务处理能力。
go get github.com/zhangzqs/gormasynctask
// 1. 定义任务实体
type MyTask struct {
gormasynctask.BaseTask
TaskID string `gorm:"primaryKey"`
Payload string
}
func (t *MyTask) TableName() string { return "my_tasks" }
// 2. 初始化服务
db := /* 初始化GORM连接 */
taskTable := gormasynctask.NewTaskTable[*MyTask](db)
service := gormasynctask.NewTaskService(
taskTable,
gormasynctask.RunnerFunc[*MyTask](func(ctx context.Context, task *MyTask, h gormasynctask.Handler) {
// 业务逻辑...
h.Done()
}),
)
// 3. 提交任务
taskTable.Create(context.Background(), &MyTask{
BaseTask: gormasynctask.BaseTask{TaskState: gormasynctask.TaskStateInit},
TaskID: "order-123",
})
// 4. 启动任务消费
go service.Start(context.Background(), gormasynctask.DoInput{
Limit: 100,
Concurrency: 20,
})
stateDiagram-v2
[*] --> INIT
INIT --> DOING: 任务获取
DOING --> DONE: 处理成功
DOING --> ERROR: 处理失败
DOING --> PENDING: 延迟重试
PENDING --> DOING: 到达延迟时间
ERROR --> INIT: 手动重置
type Handler interface {
// 标记成功(无额外字段更新)
Done()
// 标记成功并更新字段
DoneWithUpdater(updater map[string]any)
// 延迟执行(默认字段更新)
Pending(delayTime time.Duration)
// 延迟执行并更新字段
PendingWithUpdater(updater map[string]any, delayTime time.Duration)
// 标记失败
Error(err error)
}
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.