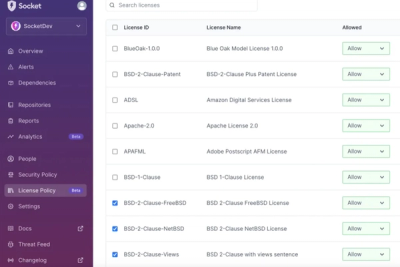
Product
Introducing License Enforcement in Socket
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
com.github.krenfro:eztexting-java
Advanced tools
A Java client for the EZ Texting SMS text messaging API
A Java client for the EZ Texting SMS text messaging API
EZ Texting provides an API for sending SMS text messages.
You will need an account to use this library. Consult the API Documentation
This library does not implement all the features of their API.
This library can:
This library cannot:
If you need a feature implemented, please submit a pull request or file an issue.
EzTextingClient ez = new EzTextingClient(
new EzTextingCredentials("username","password"));
Messaging messaging = new Messaging(ez);
Message message = new Message.Builder()
.subject("test")
.message("Here I am EZ Texting")
.phone("5551234567")
.build();
messaging.send(message);
GroupManager groups = new GroupManager(ez);
Group group = groups.create("neat");
GroupQuery query = new GroupQuery.Builder()
.sortBy(SortBy.GROUP_NAME)
.itemsPerPage(10)
.page(1)
.build();
List<Group> all = groups.retrieveAll(query);
ContactManager contacts = new ContactManager(ez);
CreateRequest request = new CreateRequest.Builder()
.phone("5551234567")
.first("first")
.last("last")
.email("my@email.com")
.group("group1")
.group("group2")
.build();
Contact contact = contacts.create(request);
UpdateRequest update = new UpdateRequest.Builder()
.email("another@email.com")
.group("group1", "group3")
.build();
contact = contacts.update(contact, update);
contacts.delete(contact);
ContactQuery query = new ContactQuery.Builder()
.query("5551234567")
.sortBy(SortBy.LAST_NAME)
.ascending()
.itemsPerPage(100)
.build();
List<Contact> found = contactManager.retrieveAll(query);
This library (will soon) be in the Maven Central Repository.
<dependencies>
<dependency>
<groupId>com.github.krenfro</groupId>
<artifactId>eztexting-java</artifactId>
<version>0.0.1</version>
</dependency>
</dependencies>
FAQs
A Java client for the EZ Texting SMS text messaging API
We found that com.github.krenfro:eztexting-java demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.