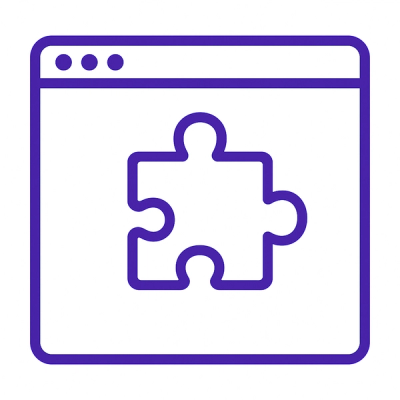
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
@acdibble/lazy-range
Advanced tools
range()
in JSThis is a memory-efficient range class. It only stores the start, stop, step,
and length for a range. The values are generated as necessary via
Symbol.iterator
. It works with any ES2015 compliant browsers.
Additionally, it's been adapted to match expected JavaScript behavior, e.g.
using LazyRange#at
to look up an out of bounds index will return undefined, rather
than throwing an error.
const LazyRange = require('@acdibble/lazy-range');
or with modules:
import LazyRange from '@acdibble/lazy-range';
>>> list(range(10))
[0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
> [...new LazyRange(10)];
[ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 ]
> Array.from(new LazyRange(10));
[ 0, 1, 2, 3, 4, 5, 6, 7, 8, 9 ]
>>> list(range(-10, 0))
[-10, -9, -8, -7, -6, -5, -4, -3, -2, -1]
> [...new LazyRange(-10, 0)];
[ -10, -9, -8, -7, -6, -5, -4, -3, -2, -1 ]
>>> list(range(1, 100, 25))
[1, 26, 51, 76]
> [...new LazyRange(1, 100, 25)];
[ 1, 26, 51, 76 ]
>>> sum = 0
>>> for x in range(10):
... sum += x
...
>>> sum
45
> let sum = 0;
undefined
> for (const x of new LazyRange(10)) {
... sum += x;
... }
45
or
> [...new LazyRange(10)].reduce((acc, num) => acc + num, 0);
45
range(0, 3, 2) == range(0, 4, 2)
True
> new LazyRange(0, 3, 2).equals(new LazyRange(0, 4, 2))
true
>>> range(0, 20, 2)[4:-3:2]
range(8, 14, 4)
> new LazyRange(0, 20, 2).slice(4, -3, 2);
LazyRange { start: 8, step: 4, stop: 14, length: 2 }
>>> len(range(10))
10
> new LazyRange(10).length;
10
>>> 10 in range(0, 20, 2)
True
> new LazyRange(0, 20, 2).has(10);
true
>>> range(1, 19, 3)[5]
16
> new LazyRange(1, 19, 3).at(5);
16
>>> range(1, 19, 3).index(13)
4
> new LazyRange(1, 19, 3).indexOf(13);
4
FAQs
A port of Python 3's range class to JavaScript.
We found that @acdibble/lazy-range demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.