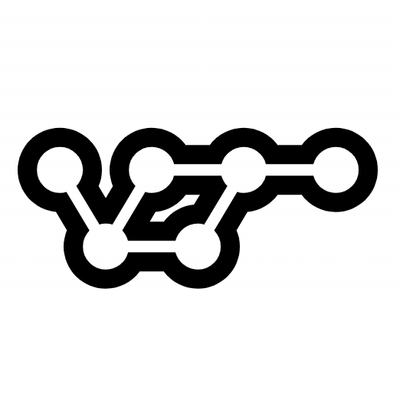
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@aerisweather/deploy-lambda-function
Advanced tools
Helper for deploying code to AWS Lambda.
zip
file (excludes devDependencies, to keep it small)Install via npm:
npm install --save-dev @aerisweather/deploy-lambda-function
First, you'll need to setup a param.json
config files for each deployment environment. For example:
// params.staging.json
{
// List of directories to include in `zip` file
"srcDirs": ["dist/lib", "node_modules"],
// Name of your lambda function on AWS
"lambdaFunction": "my-lambda-function",
// Location of environment variables on S3
"envFile": "s3://my-bucket/env/staging.env",
// The alias you want to point at your lambda function
"lambdaAlias": "staging",
// The IAM role you want to give your lambda function
"lambdaRole": "arn:aws:iam::ACCOUNT_ID:role/my-lambda-function-staging",
// The region associated with your lambda function (optional, defaults to us-east-1)
"lambdaRegion": "us-east-1"
}
To deploy to that environment, run:
node_modules/.bin/deploy-lambda-function -c ./params.staging.json
You can also use this library programmatically, via a node script:
const deployLambdaFunction = require('@aerisweather/deploy-lambda-function').default;
deployLambdaFunction({
srcDirs: ['dist/lib', 'node_modules'],
lambdaFunction: 'my-lambda-function',
envFile: 's3://my-bucket/env/staging.env',
lambdaAlias: 'staging',
lambdaRole: 'arn:aws:iam::ACCOUNT_ID:role/my-lambda-function-staging',
lambdaRegion: 'us-east-1'
})
You can setup BitBucket pipelines to deploy to lambda, using deploy-lambda-function
.
For this to work, you'll need to:
params.json
files for prod
, staging
, and dev
bitbucket-pipelines.yml
file below to your repo# bitbucket-pipelines.yml
# Run on LambCI's lambda-simulation container,
# to give an environment that's close to the real deal
image: lambci/build-nodejs4.3
clone:
depth: 1
pipelines:
# Default build scripts...
default:
- step:
script:
# Configure npm with your NPM_TOKEN
# (only required if installing from a private npm registry)
- touch .npmrc && echo "//registry.npmjs.org/:_authToken=${NPM_TOKEN}" >> .npmrc
# Build and test your code
- npm install -g npm
- npm install
- npm run build
- npm run test
# master/staging/development get deployed to lambda
branches:
master:
- step:
script:
# Configure npm with your NPM_TOKEN
# (only required if installing from a private npm registry)
- touch .npmrc && echo "//registry.npmjs.org/:_authToken=${NPM_TOKEN}" >> .npmrc
# Build your code
- npm install && npm build && npm test
# Deploy to lambda, using prod config
- ./node_modules/.bin/deploy-lambda-function -c ./deploy/params.prod.json
staging:
- step:
script:
# Configure npm with your NPM_TOKEN
# (only required if installing from a private npm registry)
- touch .npmrc && echo "//registry.npmjs.org/:_authToken=${NPM_TOKEN}" >> .npmrc
# Build your code
- npm install && npm build && npm test
# Deploy to lambda, using staging config
- ./node_modules/.bin/deploy-lambda-function -c ./deploy/params.staging.json
development:
- step:
script:
# Configure npm with your NPM_TOKEN
# (only required if installing from a private npm registry)
- touch .npmrc && echo "//registry.npmjs.org/:_authToken=${NPM_TOKEN}" >> .npmrc
# Build your code
- npm install && npm build && npm test
# Deploy to lambda, using dev config
- ./node_modules/.bin/deploy-lambda-function -c ./deploy/params.dev.json
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Action": [
"lambda:PublishVersion",
"lambda:UpdateAlias",
"lambda:UpdateFunctionCode",
"lambda:UpdateFunctionConfiguration"
],
"Resource": [
"arn:aws:lambda:ACCOUNT_REGION:ACCOUNT_ID:function:NAME_OF_YOUR_LAMBDA_FUNCTION"
]
}
]
}
~/.npmrc
to the NPM_TOKEN
env var in Pipelines
FAQs
Script for deploying code as a lambda function
We found that @aerisweather/deploy-lambda-function demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.