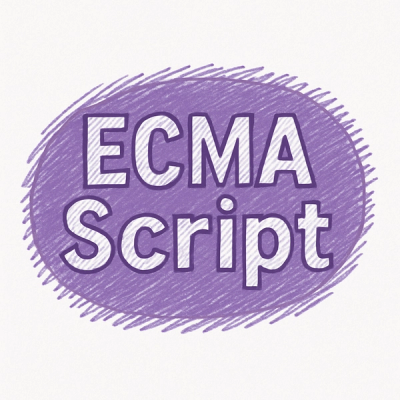
Security News
ECMAScript 2025 Finalized with Iterator Helpers, Set Methods, RegExp.escape, and More
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
@algolia/autocomplete-js
Advanced tools
Fast and fully-featured autocomplete JavaScript library.
The autocomplete-js
package is an agnostic virtual DOM renderer. You can use it in JavaScript, Preact, React, or Vue projects.
yarn add @algolia/autocomplete-js
# or
npm install @algolia/autocomplete-js
See Documentation.
FAQs
Fast and fully-featured autocomplete JavaScript library.
The npm package @algolia/autocomplete-js receives a total of 119,052 weekly downloads. As such, @algolia/autocomplete-js popularity was classified as popular.
We found that @algolia/autocomplete-js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 92 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.
Research
North Korean threat actors linked to the Contagious Interview campaign return with 35 new malicious npm packages using a stealthy multi-stage malware loader.