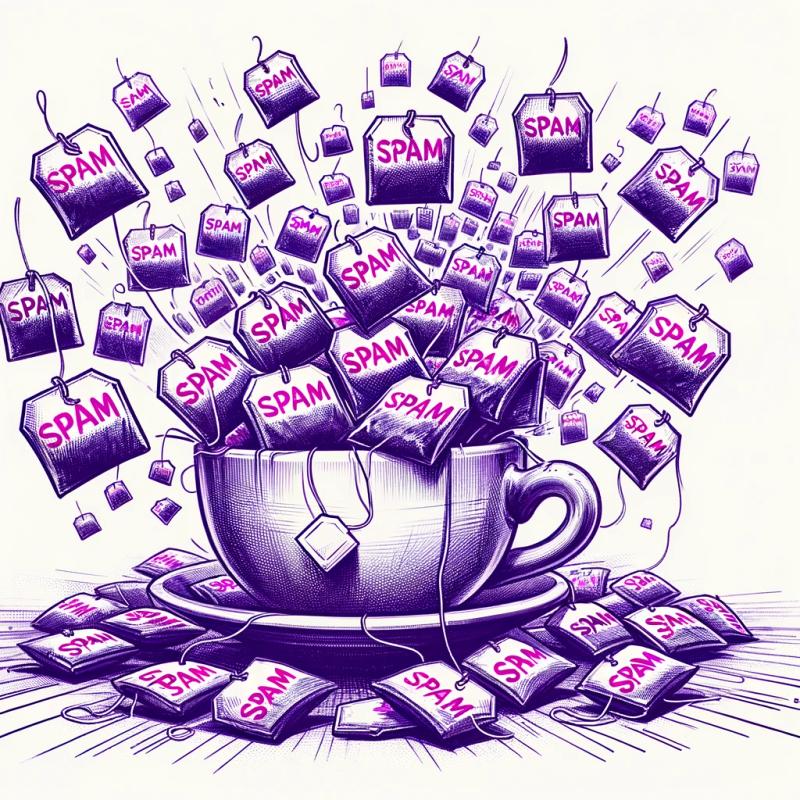
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@art4/reduxio
Advanced tools
Readme
Treat actions as they were events.
Lightweight Redux middleware that simplifies creating real-time apps with socket.io.
npm i @art4/reduxio
import { createStore, applyMiddleware } from 'redux';
import io from 'socket.io-client';
import { createIoMiddleware } from '@art4/reduxio';
const socket = io('localhost');
const ioMiddleware = createIoMiddleware({
socket,
/* Listen to events (action types) that are going to be automatically dispatched to the store. */
listenTo: ['MESSAGE_RECEIVE']
});
const store = createStore(
reducers,
applyMiddleware(ioMiddleware)
);
import { createStore, applyMiddleware } from 'redux';
import io from 'socket.io-client';
import { createIoMiddleware } from '@art4/reduxio';
const socket = io('localhost');
const ioMiddleware = createIoMiddleware({
socket,
listenTo: ['$_MESSAGE_RECEIVE']
});
const store = createStore(
reducers,
applyMiddleware(ioMiddleware)
);
store.dispatch({
type: 'MESSAGE_SEND',
payload: 'Message sent from client'
});
socket.on('MESSAGE_SEND', (action, dispatchOnce) => {
/* Emitting an action to connected clients, except the sender. */
socket.emit('$_MESSAGE_RECEIVE', {
type: '$_MESSAGE_RECEIVE',
payload: action.payload
});
/*
We are allowed to dispatch one action to the sender using the helper.
Obviously, dispatching more actions is available through emit.
Advantage of this approach is that we don't have to set up a listener for this action type.
*/
dispatchOnce({ type: '$_MESSAGE_SUCCESS' });
});
Creates redux middleware with options.
Options:
Name | Type | Default | Required | Description |
---|---|---|---|---|
socket | Object | yes | Socket.io client instance. | |
autoEmit | Boolean | true | Automatically emit dispatched actions. Can be overwritten for specific action with meta io: false option. | |
listenTo | Array | [] | Action types (event names) that are going to be automatically dispatched to the store. |
Options that are passed to action's meta as io
property.
io: boolean Determines if the action has to be emitted or not.
io: object Allows to pass options when emitting specific action.
Name | Type | Default | Description |
---|---|---|---|
withState | Boolean | false | Emits action with current store state (after this action has been dispatched). |
Client
store.dispatch({
type: 'MESSAGE_SEND',
payload: 'Hello',
meta: { io: { withState: true }}
});
Server
socket.on('MESSAGE_SEND', (action, state, dispatchOnce) => {
/*
Client's state is now available under the second argument.
Keep in mind that dispatchOnce is always provided as last argument.
*/
});
const ioMiddleware = createIoMiddleware({
socket,
autoEmit: true
});
const store = createStore(
reducers,
applyMiddleware(ioMiddleware)
);
store.dispatch({
type: 'MESSAGE_SEND',
payload: 'Hello',
meta: {
/* Auto emit option from middleware creator has lower priority, so this action won't be emitted. */
io: false
}
});
FAQs
Treat actions as they were events
The npm package @art4/reduxio receives a total of 1 weekly downloads. As such, @art4/reduxio popularity was classified as not popular.
We found that @art4/reduxio demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.