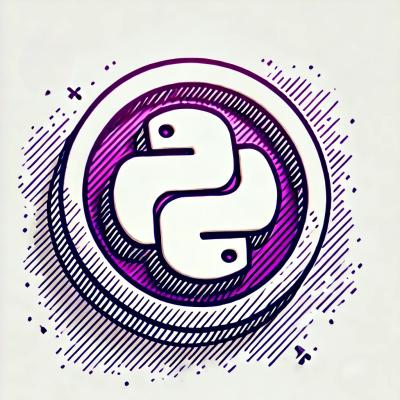
Security News
PyPI Now Supports iOS and Android Wheels for Mobile Python Development
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
@automatic-labs/mustang-navigation
Advanced tools
The Mustang Navigation API has methods available for Automatic platform app developers to provide an integrated user experience in the context of Automatic's native iOS and Android apps.
npm install @automatic-labs/mustang-navigation --save
import navigation from '@automatic-labs/mustang-navigation'
You can query the navigation API to understand more about the current application context.
Type: string|null
The current Automatic vehicleID
context that your web app is in. This is useful if you want to present vehicle specific content. See the pushView
and presentModal
methods to see how to change the vehicleID
for subsequent views.
request.get(`http://your-api.com/vehicles/${navigation.vehicleID}`, (err, res) => {
if (!err) {
console.log('Vehicle specific response', res);
}
});
Type: string
The operating system or platform the Automatic app is running on.
Your webapp may want to know what operating system the Automatic app is being run on. We have created a convenient property that allows you to query this.
With version 1.3.0 and above you should use the PLATFORM enum to check against a platform.
import navigation, { PLATFORM } from '@automatic-labs/mustang-navigation';
if (navigation.platform === PLATFORM.ANDROID)
console.log('This is an Android device')
else if (navigation.platform === PLATFORM.IOS)
console.log('This is an iOS device')
else if (navigation.platform === PLATFORM.WEB)
console.log('This is probably in a web browser')
else
console.log('This is some unknown device type')
Pushes should be used to add a new view to the Automatic app's navigation stack when you want the user to dig deeper into a given context. The new view will have a "back button" that will return the user to the previous view.
Pushes a url onto the navigation stack. The state of the previous view is generally retained, but this is not guaranteed (eg. can be cleared if the app is backgrounded).
A relative path
or absolute url
may be pushed.
Object: { path, url }
navigation.pushView({ path: '/#/some-record-name' })
Pushes the Check Engine Light view for a specified that vehicle onto the navigation stack. This is a native view.
String
navigation.pushCheckEngineLight('C_IGA217362875213')
Modals should be used when you want the user to perform an action, such as selection or input from a given context. They appear on top of the view they are presented from, and dismiss back to that view.
A user can always exit a modal back to the presenting view by pressing the "Exit" action.
Presents a modal view over the current view. Can present either a relative path
, or absolute url
. A title
should be given so it can be referred to if the user wants to exit.
Object: { title, path, url }
null|Object|String|Number|Array|Boolean
dismissModal
is called from the modal view with a payload
. Can be . Will be null
if no payload is sent.navigation.presentModal({
title: 'Adjust a value',
path: '/#/some-input-path'
}, (payload) => {
if (payload !== null) {
console.log('A payload was received from the modal', payload)
}
})
Only relevant if a view is in a modal context. Dismisses the modal view to the launching view. Can dismiss with a payload
which will be received by the launching view on the modalDidDismiss
event.
null|Object|String|Number|Array|Boolean
navigation.dismissModal({
value: 128761,
optionSelected: true,
optionValues: ['Audi', 'Volkwagen', 'Porsche']
})
Your app may need to launch external apps or websites. But in order to do so safely, you must first check whether the Automatic app supports opening a given app by checking if its url pattern can be used to launch the app. UI to open these apps/urls should only be presented if the url action is supported.
Used to test whether the host device can handle various urls. This is useful if you'd like to test whether an application is installed, or the type of url to use for handling types of information (eg. the url prefix for maps is very different between Google Maps on iOS vs Android, and Apple maps on iOS.)
The applicationCanOpenExternalURL
event will be asynchronously called when the native Automatic app determines whether it can open the given url.
String
id
to give this particular url pattern.String
url
to test.Boolean
url
can be opened on from the Automatic app.const urls = {
get googleMaps() {
const mapsUrlScheme = navigation.OS === 'iOS' ? 'comgooglemaps://' : 'geo://'
return `${mapsUrlScheme}37.7619803,-122.4128065`
},
appleMaps: 'maps://?q=Mexican+Restaurant',
nest: 'nest://'
}
navigation.canOpenExternalURL(urls.appleMaps, (canOpen) => {
if (canOpen) console.log('Can open Apple Maps')
})
navigation.canOpenExternalURL(urls.googleMaps, (canOpen) => {
if (canOpen) console.log('Can open Google Maps')
})
navigation.canOpenExternalURL(urls.nest, (canOpen) => {
if (canOpen) console.log('Can open Nest')
})
Opens in the provided url in an external application that is registered to handle it. Should only be called after proper checking with canOpenExternalURL
.
String
url
to test.navigation.on('applicationCanOpenExternalURL', (canOpen, id, url) => {
if (canOpen && id === 'hasGoogleMaps') {
console.log('Opening google maps')
navigation.openExternalURL(url)
}
})
In order to ensure that users do not confuse points of failure of any technical issues your app may be experiencing, you must use the presentAPIErrorMessage
when a request to your app's API fails..
The only exception is if your request error is a 401
authorization error. In this case you should restart the auth flow.
Used to present a predefined error message to the user about your app failing. An optional callback will be called when when the user dismisses the error message.
Used in the context of a request:
request.get('https://your-api.com/invalid', (err, res) => {
if (err) {
if(err.status !== 401) {
navigation.presentAPIErrorMessage();
} else {
restartYourAuthFlow();
}
} else {
doSomethingGood();
}
});
This will show a web alert with the following copy when the request returns a non-401 error:
This service experienced an error making a request. This may be due to an issue with its servers. Please try again in a bit.
FAQs
Automatic Mustang Navigation API
We found that @automatic-labs/mustang-navigation demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.