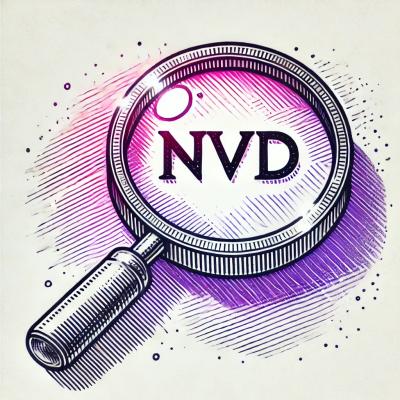
Security News
NIST Under Federal Audit for NVD Processing Backlog and Delays
As vulnerability data bottlenecks grow, the federal government is formally investigating NIST’s handling of the National Vulnerability Database.
@aws-crypto/material-management
Advanced tools
@aws-crypto/material-management is a package provided by AWS that offers tools for managing cryptographic materials. It is part of the AWS Encryption SDK and is used to handle encryption keys and cryptographic operations in a secure and efficient manner.
Keyring Management
This feature allows you to create and manage keyrings, which are collections of cryptographic keys used for encryption and decryption. The code sample demonstrates how to create a KMS keyring using AWS KMS keys.
const { KmsKeyringNode } = require('@aws-crypto/client-node');
const keyring = new KmsKeyringNode({
generatorKeyId: 'arn:aws:kms:us-west-2:123456789012:key/abcd-1234-efgh-5678',
keyIds: ['arn:aws:kms:us-west-2:123456789012:key/ijkl-9012-mnop-3456']
});
Cryptographic Materials Management
This feature provides tools for managing cryptographic materials, including encryption and decryption contexts. The code sample shows how to create a client with a specified keyring and encryption context.
const { getClient } = require('@aws-crypto/client-node');
const client = getClient({
keyring,
encryptionContext: { purpose: 'example' }
});
Encryption and Decryption
This feature allows you to perform encryption and decryption operations using the managed cryptographic materials. The code sample demonstrates how to encrypt and decrypt data using a keyring.
const { encrypt, decrypt } = require('@aws-crypto/client-node');
const plaintext = 'Hello, World!';
async function encryptData() {
const { result } = await encrypt(keyring, plaintext);
return result;
}
async function decryptData(ciphertext) {
const { plaintext } = await decrypt(keyring, ciphertext);
return plaintext;
}
node-forge is a JavaScript library that provides a wide range of cryptographic tools, including key generation, encryption, and decryption. It is more general-purpose compared to @aws-crypto/material-management, which is specifically designed for integration with AWS services.
crypto-js is a popular library for cryptographic operations in JavaScript. It offers various algorithms for hashing, encryption, and decryption. Unlike @aws-crypto/material-management, crypto-js does not provide built-in support for AWS KMS or key management features.
Stanford JavaScript Crypto Library (sjcl) is a library for cryptographic operations in JavaScript. It focuses on providing secure and efficient cryptographic primitives. While it offers similar functionalities, it lacks the AWS-specific integrations found in @aws-crypto/material-management.
The AWS Encryption SDK for JavaScript is a client-side encryption library designed to make it easy for everyone to encrypt and decrypt data using industry standards and best practices. It uses a data format compatible with the AWS Encryption SDKs in other languages. For more information on the AWS Encryption SDKs in all languages, see the Developer Guide.
This package is not intended for direct use by clients. To get started with the AWS Encryption SDK for JavaScript, follow the instructions in the README.
This SDK is distributed under the Apache License, Version 2.0, see LICENSE.txt and NOTICE.txt for more information.
FAQs
Unknown package
The npm package @aws-crypto/material-management receives a total of 264,192 weekly downloads. As such, @aws-crypto/material-management popularity was classified as popular.
We found that @aws-crypto/material-management demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 8 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
As vulnerability data bottlenecks grow, the federal government is formally investigating NIST’s handling of the National Vulnerability Database.
Research
Security News
Socket’s Threat Research Team has uncovered 60 npm packages using post-install scripts to silently exfiltrate hostnames, IP addresses, DNS servers, and user directories to a Discord-controlled endpoint.
Security News
TypeScript Native Previews offers a 10x faster Go-based compiler, now available on npm for public testing with early editor and language support.