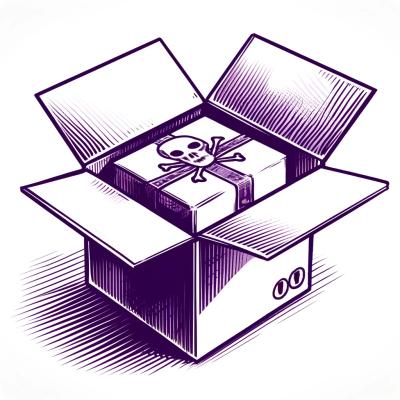
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
@aws-sdk/client-cloudwatch
Advanced tools
AWS SDK for JavaScript Cloudwatch Client for Node.js, Browser and React Native
@aws-sdk/client-cloudwatch is an AWS SDK for JavaScript package that allows developers to interact with Amazon CloudWatch, a monitoring and observability service. It provides functionalities to collect and track metrics, collect and monitor log files, and set alarms.
PutMetricData
This feature allows you to publish metric data points to Amazon CloudWatch. The code sample demonstrates how to send a custom metric 'PageViewCount' with a dimension 'PageURL' to the 'MyApp' namespace.
const { CloudWatchClient, PutMetricDataCommand } = require('@aws-sdk/client-cloudwatch');
const client = new CloudWatchClient({ region: 'us-west-2' });
const params = {
MetricData: [
{
MetricName: 'PageViewCount',
Dimensions: [
{
Name: 'PageURL',
Value: 'example.com/home'
}
],
Unit: 'Count',
Value: 1
}
],
Namespace: 'MyApp'
};
const run = async () => {
try {
const data = await client.send(new PutMetricDataCommand(params));
console.log('Success', data);
} catch (err) {
console.error('Error', err);
}
};
run();
GetMetricData
This feature allows you to retrieve metric data from Amazon CloudWatch. The code sample demonstrates how to get the average CPU utilization for a specific EC2 instance over the past hour.
const { CloudWatchClient, GetMetricDataCommand } = require('@aws-sdk/client-cloudwatch');
const client = new CloudWatchClient({ region: 'us-west-2' });
const params = {
MetricDataQueries: [
{
Id: 'm1',
MetricStat: {
Metric: {
Namespace: 'AWS/EC2',
MetricName: 'CPUUtilization',
Dimensions: [
{
Name: 'InstanceId',
Value: 'i-1234567890abcdef0'
}
]
},
Period: 300,
Stat: 'Average'
},
ReturnData: true
}
],
StartTime: new Date(Date.now() - 3600 * 1000),
EndTime: new Date()
};
const run = async () => {
try {
const data = await client.send(new GetMetricDataCommand(params));
console.log('Success', data);
} catch (err) {
console.error('Error', err);
}
};
run();
PutDashboard
This feature allows you to create or update a CloudWatch dashboard. The code sample demonstrates how to create a dashboard named 'MyDashboard' with a widget that displays the average CPU utilization of a specific EC2 instance.
const { CloudWatchClient, PutDashboardCommand } = require('@aws-sdk/client-cloudwatch');
const client = new CloudWatchClient({ region: 'us-west-2' });
const params = {
DashboardName: 'MyDashboard',
DashboardBody: JSON.stringify({
widgets: [
{
type: 'metric',
x: 0,
y: 0,
width: 24,
height: 6,
properties: {
metrics: [
['AWS/EC2', 'CPUUtilization', 'InstanceId', 'i-1234567890abcdef0']
],
period: 300,
stat: 'Average',
region: 'us-west-2',
title: 'EC2 Instance CPU'
}
}
]
})
};
const run = async () => {
try {
const data = await client.send(new PutDashboardCommand(params));
console.log('Success', data);
} catch (err) {
console.error('Error', err);
}
};
run();
The 'cloudwatch' npm package is a lightweight alternative for sending custom metrics to Amazon CloudWatch. It is simpler and more focused on metric data, but lacks the comprehensive features of @aws-sdk/client-cloudwatch, such as dashboard management and alarm creation.
The 'aws-sdk' npm package is the predecessor to the modular AWS SDK v3 packages like @aws-sdk/client-cloudwatch. It provides a broader set of AWS services in a single package, but is less modular and can result in larger bundle sizes compared to the more focused v3 packages.
AWS SDK for JavaScript CloudWatch Client for Node.js, Browser and React Native.
Amazon CloudWatch monitors your Amazon Web Services (Amazon Web Services) resources and the applications you run on Amazon Web Services in real time. You can use CloudWatch to collect and track metrics, which are the variables you want to measure for your resources and applications.
CloudWatch alarms send notifications or automatically change the resources you are monitoring based on rules that you define. For example, you can monitor the CPU usage and disk reads and writes of your Amazon EC2 instances. Then, use this data to determine whether you should launch additional instances to handle increased load. You can also use this data to stop under-used instances to save money.
In addition to monitoring the built-in metrics that come with Amazon Web Services, you can monitor your own custom metrics. With CloudWatch, you gain system-wide visibility into resource utilization, application performance, and operational health.
To install this package, simply type add or install @aws-sdk/client-cloudwatch using your favorite package manager:
npm install @aws-sdk/client-cloudwatch
yarn add @aws-sdk/client-cloudwatch
pnpm add @aws-sdk/client-cloudwatch
The AWS SDK is modulized by clients and commands.
To send a request, you only need to import the CloudWatchClient
and
the commands you need, for example ListMetricsCommand
:
// ES5 example
const { CloudWatchClient, ListMetricsCommand } = require("@aws-sdk/client-cloudwatch");
// ES6+ example
import { CloudWatchClient, ListMetricsCommand } from "@aws-sdk/client-cloudwatch";
To send a request, you:
send
operation on client with command object as input.destroy()
to close open connections.// a client can be shared by different commands.
const client = new CloudWatchClient({ region: "REGION" });
const params = {
/** input parameters */
};
const command = new ListMetricsCommand(params);
We recommend using await operator to wait for the promise returned by send operation as follows:
// async/await.
try {
const data = await client.send(command);
// process data.
} catch (error) {
// error handling.
} finally {
// finally.
}
Async-await is clean, concise, intuitive, easy to debug and has better error handling as compared to using Promise chains or callbacks.
You can also use Promise chaining to execute send operation.
client.send(command).then(
(data) => {
// process data.
},
(error) => {
// error handling.
}
);
Promises can also be called using .catch()
and .finally()
as follows:
client
.send(command)
.then((data) => {
// process data.
})
.catch((error) => {
// error handling.
})
.finally(() => {
// finally.
});
We do not recommend using callbacks because of callback hell, but they are supported by the send operation.
// callbacks.
client.send(command, (err, data) => {
// process err and data.
});
The client can also send requests using v2 compatible style. However, it results in a bigger bundle size and may be dropped in next major version. More details in the blog post on modular packages in AWS SDK for JavaScript
import * as AWS from "@aws-sdk/client-cloudwatch";
const client = new AWS.CloudWatch({ region: "REGION" });
// async/await.
try {
const data = await client.listMetrics(params);
// process data.
} catch (error) {
// error handling.
}
// Promises.
client
.listMetrics(params)
.then((data) => {
// process data.
})
.catch((error) => {
// error handling.
});
// callbacks.
client.listMetrics(params, (err, data) => {
// process err and data.
});
When the service returns an exception, the error will include the exception information, as well as response metadata (e.g. request id).
try {
const data = await client.send(command);
// process data.
} catch (error) {
const { requestId, cfId, extendedRequestId } = error.$metadata;
console.log({ requestId, cfId, extendedRequestId });
/**
* The keys within exceptions are also parsed.
* You can access them by specifying exception names:
* if (error.name === 'SomeServiceException') {
* const value = error.specialKeyInException;
* }
*/
}
Please use these community resources for getting help. We use the GitHub issues for tracking bugs and feature requests, but have limited bandwidth to address them.
aws-sdk-js
on AWS Developer Blog.aws-sdk-js
.To test your universal JavaScript code in Node.js, browser and react-native environments, visit our code samples repo.
This client code is generated automatically. Any modifications will be overwritten the next time the @aws-sdk/client-cloudwatch
package is updated.
To contribute to client you can check our generate clients scripts.
This SDK is distributed under the Apache License, Version 2.0, see LICENSE for more information.
FAQs
AWS SDK for JavaScript Cloudwatch Client for Node.js, Browser and React Native
We found that @aws-sdk/client-cloudwatch demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.