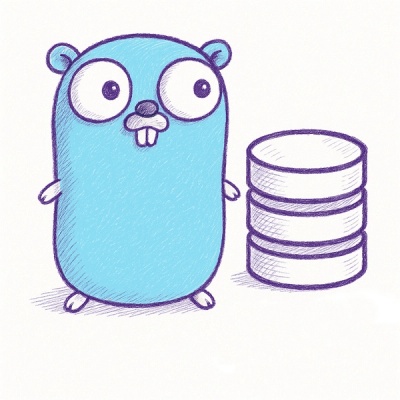
Security News
Official Go SDK for MCP in Development, Stable Release Expected in August
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
@brainstack/inject
Advanced tools
@brainstack/inject
is a lightweight yet robust dependency injection (DI) library designed for JavaScript and TypeScript projects. It simplifies dependency management, promotes code reusability, and enhances testability. This library is particularly well-suited for projects with complex dependency graphs, including monorepo architectures, providing support for various service scopes and simplifying the process of dependency injection.
@Service
, @SingletonService
, and @Inject
for simplified service creation and registration.npm install @brainstack/inject
import { Container, Service, Inject } from '@brainstack/inject';
// Create a container
const container = new Container();
// Define a service
@Service()
class DatabaseService {
getData() {
return 'Data from database';
}
}
// Define a class that depends on the service
@Service() // MyClass is also a service, so can be injected elsewhere
class MyClass {
constructor(@Inject private dbService: DatabaseService) {}
doSomething() {
const data = this.dbService.getData();
console.log(data); // Output: 'Data from database'
}
}
// Get an instance of MyClass with its dependencies injected
const myInstance = container.getInstance(MyClass);
myInstance.doSomething();
import { SingletonService } from '@brainstack/inject';
@SingletonService
class LoggerService {
// ...
}
container.register('myTransientService', () => new MyTransientService(), true); // true for transient
// Accessing the transient service
const transientServiceFactory = container.get('myTransientService');
const transientInstance1 = transientServiceFactory(); // New instance
const transientInstance2 = transientServiceFactory(); // Another new instance
asScopedService
to ensure it gets registered to only a particular container.import { asScopedService } from '@brainstack/inject';
const scopedContainer = new Container();
const ScopedService = asScopedService(MyService, scopedContainer);
const scopedInstance = scopedContainer.getInstance(ScopedService);
register(identifier, instanceOrFactory, transient?)
: Registers a service with the container.get(identifier)
: Retrieves a service from the container. Returns undefined
if not found.getInstance(ctor)
: Resolves dependencies and creates an instance of the given class (constructor injection).reset()
: Removes all registered services (useful for testing).getRegisteredServiceIdentifiers()
: Returns an array of registered service identifiers.You can create parent-child container relationships to manage dependencies across different parts of your application.
const parentContainer = new Container();
const childContainer = new Container();
@Service(parentContainer) // Make AuthService available to child containers
class AuthService {
/* ... */
}
// Register a service specific to the child container
@Service(childContainer)
class ChildService {
constructor(@Inject private authService: AuthService) {} // Injected from parent
}
const childInstance = childContainer.getInstance(ChildService); // authService injected
import {
Container,
Service,
Inject,
SingletonService,
asService,
} from '@brainstack/inject';
const container = new Container();
class BaseLogger {
log(m: string) {
console.log(m);
}
}
const LoggerService = asSingletonService(BaseLogger);
@Service()
class DatabaseService {
getData() {
return 'data!';
}
}
@Service()
class MyService {
constructor(
@Inject private database: DatabaseService,
@Inject private logger: LoggerService
) {}
doWork() {
this.logger.log('doing work');
return this.database.getData();
}
}
const myService = container.getInstance(MyService); // logger and database injected
myService.doWork(); // Output: doing work, then data from database
This enhanced documentation provides more comprehensive explanations, clearer code examples, and a better structure to help developers effectively utilize the @brainstack/inject
library. It highlights the library's key features, including its helper functions, hierarchical DI support, and various service scopes, making it a valuable resource for both novice and experienced developers.
FAQs
A Micro Dependency Injection Package
The npm package @brainstack/inject receives a total of 228 weekly downloads. As such, @brainstack/inject popularity was classified as not popular.
We found that @brainstack/inject demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.