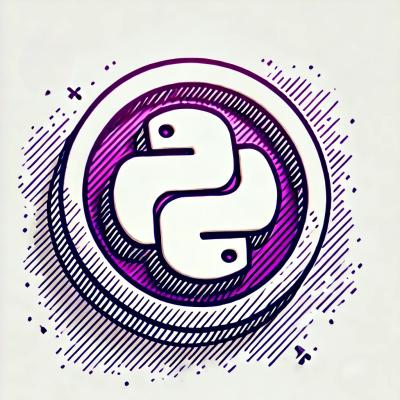
Security News
PyPI Now Supports iOS and Android Wheels for Mobile Python Development
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
@braintree/wrap-promise
Advanced tools
@braintree/wrap-promise is a utility library that helps in converting callback-based functions into promise-based ones. This is particularly useful for modernizing codebases and making asynchronous code easier to read and maintain.
Wrap a callback-based function
This feature allows you to wrap a traditional callback-based function and convert it into a promise-based function. This makes it easier to work with asynchronous code using modern JavaScript syntax.
const wrapPromise = require('@braintree/wrap-promise');
function callbackFunction(arg, callback) {
setTimeout(() => {
if (arg) {
callback(null, 'Success');
} else {
callback('Error');
}
}, 1000);
}
const promiseFunction = wrapPromise(callbackFunction);
promiseFunction(true)
.then(result => console.log(result))
.catch(error => console.error(error));
Wrap a function with multiple arguments
This feature demonstrates how to wrap a function that takes multiple arguments before the callback. The wrapped function can then be used as a promise-based function.
const wrapPromise = require('@braintree/wrap-promise');
function multiArgFunction(arg1, arg2, callback) {
setTimeout(() => {
if (arg1 && arg2) {
callback(null, 'Success');
} else {
callback('Error');
}
}, 1000);
}
const promiseFunction = wrapPromise(multiArgFunction);
promiseFunction('arg1', 'arg2')
.then(result => console.log(result))
.catch(error => console.error(error));
The `util.promisify` function in Node.js standard library serves a similar purpose by converting callback-based functions to promise-based ones. It is a built-in utility and does not require an additional package, making it a convenient option for Node.js developers.
Bluebird is a fully-featured promise library that includes a `promisify` method to convert callback-based functions to promises. It offers more advanced features and better performance compared to native promises, making it a robust choice for complex applications.
Pify is a simple library that converts callback-based functions to promise-based ones. It is lightweight and easy to use, making it a good alternative for developers who need a straightforward solution without additional features.
Small module to help support APIs that return a promise or use a callback.
// my-method.js
var wrapPromise = require("wrap-promise");
function myMethod(arg) {
return new Promise(function (resolve, reject) {
doSomethingAsync(arg, function (err, response) {
if (err) {
reject(err);
return;
}
resolve(response);
});
});
}
module.exports = wrapPromise(myMethod);
// my-app.js
var myMethod = require("./my-method");
myMethod("foo")
.then(function (response) {
// do something with response
})
.catch(function (err) {
// handle error
});
myMethod("foo", function (err, response) {
if (err) {
// handle error
return;
}
// do something with response
});
function MyObject() {}
MyObject.prototype.myAsyncMethod = function () {
return new Promise(function (resolve, reject) {
//
});
};
MyObject.prototype.myOtherAsyncMethod = function () {
return new Promise(function (resolve, reject) {
//
});
};
module.exports = wrapPromise.wrapPrototype(MyObject);
Static methods, sync methods on the prototype (though if you pass a function as the last argument of your sync method, you will get an error), and non-function properties on the prototype are ignored.
If there are certain methods you want ignored, you can pass an ignoreMethods
array.
module.exports = wrapPromise.wrapPrototype(MyObject, {
ignoreMethods: ["myMethodOnThePrototypeIDoNotWantTransformed"],
});
By default, wrapPrototype
ignores methods that begin with an underscore. You can override this behavior by passing: transformPrivateMethods: true
module.exports = wrapPromise.wrapPrototype(MyObject, {
transformPrivateMethods: true,
});
3.0.2
cross-spawn
to 7.0.6micromatch
to 4.0.8FAQs
A small lib to return promises or use callbacks
The npm package @braintree/wrap-promise receives a total of 0 weekly downloads. As such, @braintree/wrap-promise popularity was classified as not popular.
We found that @braintree/wrap-promise demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.