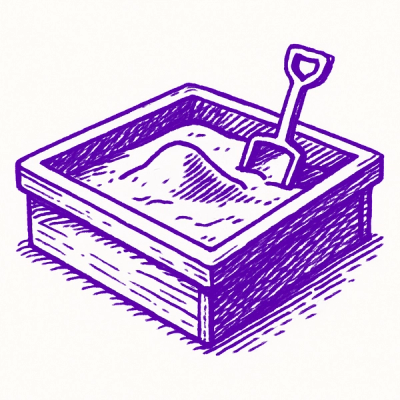
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
@brightcove/dynamodb-connector
Advanced tools
Provides a set of helpful functions for connecting to DynamoDB and performing operations
Provides a standardized way to connect to an AWS DynamoDB database using the v3 AWS javascript SDK.
npm install @brightcove/dynamodb-connector --save
const { DynamoDBConnector } = require('@brightcove/dynamodb-connector');
// Localstack configuration example
const db = new DynamoDBConnector({
clientConfig: {
endpoint: 'http://localhost:4566,
region: 'us-east-1'
}
});
...
const result = await db.query({
TableName: 'my-table',
...
});
Param | Type | Description | Required |
---|---|---|---|
clientConfig | DynamoDBClientConfig | https://docs.aws.amazon.com/AWSJavaScriptSDK/v3/latest/Package/-aws-sdk-client-dynamodb/TypeAlias/DynamoDBClientConfigType | yes |
translateConfig | TranslateConfig | https://docs.aws.amazon.com/AWSJavaScriptSDK/v3/latest/Package/-aws-sdk-lib-dynamodb/TypeAlias/TranslateConfig | no |
logger | Logger | What will be used for logging errors (ie. logger.error()). console is used by default if not specified | no |
This version includes breaking changes by requiring the DynamoDBDocument
to be passed in as an option. This drasticaly reduces the size of this package and removes all external dependencies.
import { DynamoDBDocument } from '@aws-sdk/lib-dynamodb';
import { DynamoDBClient } from '@aws-sdk/client-dynamodb';
import { DynamoDBConnector } from '@brightcove/dynamodb-connector';
const client = new DynamoDBClient({
endpoint: 'http://localhost:4566',
region: 'us-east-1'
});
const document = DynamoDBDocument.from(client);
const db = new DynamoDBConnector({ document });
...
const result = await db.query({
TableName: 'my-table',
...
});
Param | Type | Description | Required |
---|---|---|---|
document | DynamoDBDocument | https://docs.aws.amazon.com/AWSJavaScriptSDK/v3/latest/Package/-aws-sdk-lib-dynamodb/Class/DynamoDBDocument | yes |
const result = await db.query({
TableName: "CoffeeCrop",
KeyConditionExpression: "#primaryKey = :originCountry AND #sortKey > :roastDate",
ExpressionAttributeNames: {
"#primaryKey": "OriginCountry",
"#sortKey": "RoastDate",
},
ExpressionAttributeValues: {
":originCountry": "Ethiopia",
":roastDate": "2023-05-01",
},
});
console.log(result.Items); // And array of items that match the query
Including true
as the second parameter will automatically get all items if result.LastEvaluatedKey
is returned
const result = await db.query({
TableName: "CoffeeCrop",
KeyConditionExpression: "#primaryKey = :originCountry AND #sortKey > :roastDate",
ExpressionAttributeNames: {
"#primaryKey": "OriginCountry",
"#sortKey": "RoastDate",
},
ExpressionAttributeValues: {
":originCountry": "Ethiopia",
":roastDate": "2023-05-01",
},
}, true);
console.log(result.Items); // And array of items that match the query.
const result = await db.get({
TableName: "AngryAnimals",
Key: {
CommonName: "Shoebill",
}
});
console.log(result.Item); // The requested item
const result = await db.put({
TableName: "HappyAnimals",
Item: {
CommonName: "Shiba Inu",
},
});
This provides a helper method for easily creating an entire update expression
const updates = {
SET: [
{ key: '#last_login', value: new Date().toISOString() }
],
ADD: [
{ key: '#num_login_attempts', value: 5 }
],
REMOVE: [
{ key: '#not_logged_in' },
{ key: '#new_user' }
]
};
const { UpdateExpression, ExpressionAttributeValues,ExpressionAttributeNames } = db.toUpdateExpressionFields(updates);
console.log(UpdateExpression);
// SET #last_login = :last_login ADD #num_login_attempts :num_login_attempts REMOVE #not_logged_in, #new_user
console.log(ExpressionAttributeValues);
/**
{
":last_login": "2025-02-20T15:29:51.757Z",
":num_login_attempts": 5
}
*/
console.log(ExpressionAttributeNames);
/**
{
"#last_login": "last_login",
"#num_login_attempts": "num_login_attempts",
"#not_logged_in": "not_logged_in",
"#new_user": "new_user"
}
*/
const result = await db.update({
TableName: "Dogs",
Key: {
Breed: "Labrador",
},
UpdateExpression: "set Color = :color",
ExpressionAttributeValues: {
":color": "black",
},
ReturnValues: "ALL_NEW",
});
console.log(result.Attributes); // The updated item
await db.delete({
TableName: "Sodas",
Key: {
Flavor: "Cola",
},
});
const keys = [{ Flavor: "Cola" }, { Flavor: "Sprite" }];
const result = await db.batchGet("Sodas", keys);
console.log(result.Items); // An array of the requested items
const puts = [{ Flavor: "Cola" }, { Flavor: "Sprite" }]
.map(db.toPutRequest);
const deletes = [{ Flavor: "Mountain Dew" }, { Flavor: "Dr. Pepper" }]
.map((item) => db.toDeleteRequest(item, "Flavor"));
await db.batchWrite("Sodas", [ ...puts, ...deletes ]);
const items = [
{
Put: {
TableName: "HappyAnimals",
Item: {
Type: "Animal",
Breed: "Shiba Inu",
}
}
},
{
Update: {
TableName: "Dogs",
Key: {
Type: "Animal",
Breed: "Labrador",
},
UpdateExpression: "set Color = :color",
ExpressionAttributeValues: {
":color": "black",
},
ReturnValues: "ALL_NEW",
}
}
];
await db.transactWrite(items);
const items = [
{
TableName: "Dogs",
Key: {
Type: "Animal",
Breed: "Labrador",
},
},
{
TableName: "Dogs",
Key: {
Type: "Animal",
Breed: "Shiba Inu",
},
}
];
const result = await db.transactGet(items);
console.log(result); // An array of the requested items
const uuid = db.uuid();
console.log(uuid); // A random UUIDv4
FAQs
Provides a set of helpful functions for connecting to DynamoDB and performing operations
The npm package @brightcove/dynamodb-connector receives a total of 17 weekly downloads. As such, @brightcove/dynamodb-connector popularity was classified as not popular.
We found that @brightcove/dynamodb-connector demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 85 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.