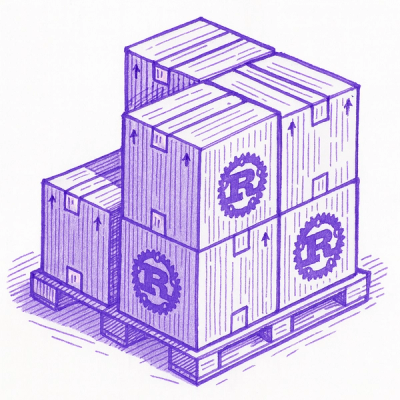
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
@btmills/queue
Advanced tools
This is a queue implementation for JavaScript with a convenient API that avoids use of the expensive (O(n)
) Array.shift()
operation.
var Queue = require('o1queue');
Create a new queue.
var q = new Queue();
Queue up some stuff.
q.enqueue('hello');
q.enqueue('world');
How big is it?
console.log(q.length); // 2
What's at the front?
console.log(q.peek()) // 'hello'
Remove some stuff.
while (q.length > 0) {
console.log(q.dequeue()) // 'hello', 'world'
}
Copyright © 2015 Brandon Mills. All rights reserved. Licensed under the MIT License, the full text of which is available in LICENSE.
FAQs
A performant queue data structure.
We found that @btmills/queue demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.