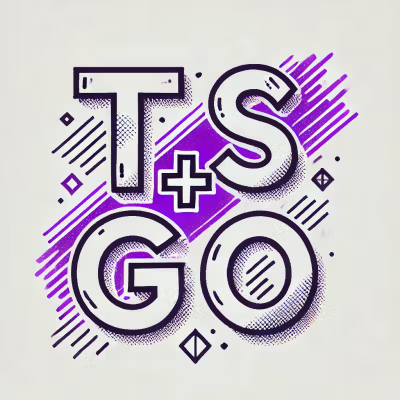
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
@byu-oit/node-global-data-manager
Advanced tools
This module originally served to store server configurations in a single location and accessing/changing them throughout the server files as necessary. I've since come across dozens of similar scenarios since that have led to the creation of this module.
With the global-data-manager, you can create, modify, or delete project-global variables in any file without the hassle of exporting and importing variables from multiple files.
This pattern makes local caching easy. Be aware on systems that use load balancing, caches between server instances will be unequal.
npm i @byu-oit/node-global-data-manager
fetch - Retrieves the key-value pair all - Retrieves all the store properties and values
put - Stores the key-value pair, over-writes the key if it already exists putMany - Stores the key-value pairs, over-writes a key if it already exists
create - Creates the key-value pair, will not over-write the key if it already exists createMany - Creates the key-value pairs, will not over-write a key if it already exists
erase - Removes the property from the global store eraseMany - Removes the properties from the global store
copy - Copy the value from one property to another
rename - Rename a property without changing the value
reset - Resets the global data store for the instance
expire - Removes the property from the store after a ttl in milliseconds expireMany - Removes the properties from the store after a ttl in milliseconds
getId - Retrieves the Manager instance identifier
(These and other examples may be found in the test directory)
const Manager = require('@byu-oit/node-global-data-manager')
const { expect } = require('chai')
const store = Manager()
const store1 = Manager('a')
const store2 = Manager('b')
const store2copy = Manager('b')
store1.put('id', 1)
store2.put('id', 2)
expect(store.getId()).to.equal(0);
expect(store1.getId()).to.equal('a')
expect(store2.getId()).to.equal('b')
expect(store.fetch('id')).to.deep.equal(undefined)
expect(store1.all()).to.deep.equal({ id: 1 })
expect(store2.all()).to.deep.equal({ id: 2 })
expect(store2copy.fetch('id')).to.deep.equal(2)
const store = require('@byu-oit/node-global-data-manager').Manager()
const { expect } = require('chai')
const toStore = {
id: 1,
name: 'Jose',
birth: new Date(),
attributes: {
hairColor: 'brn',
ethnicity: 'latin-american'
}
}
const local = store.putMany(toStore)
expect(local).to.deep.equal(toStore)
expect(store.all()).to.deep.equal(toStore)
const item = store.put('id', 2)
expect(item).to.deep.equal({id: 2})
expect(store.fetch('id')).to.equal(2)
const store = require('@byu-oit/node-global-data-manager').Manager()
const { expect } = require('chai')
const toStore = {
id: 1,
name: 'Jose',
birth: new Date(),
attributes: {
hairColor: 'brn',
ethnicity: 'latin-american'
}
}
const result1 = store.createMany(toStore)
const result2 = store.createMany(toStore)
expect(result1).to.deep.equal(toStore)
expect(store.all()).to.deep.equal(toStore)
const keys = Object.keys(result2)
expect(keys.length).to.equal(4)
keys.map(key => {
expect(result2[key]).to.equal(null)
})
const store = require('@byu-oit/node-global-data-manager').Manager()
const { expect } = require('chai')
const toStore = {
id: 1,
name: 'Jose',
birth: new Date(),
attributes: {
hairColor: 'brn',
ethnicity: 'latin-american'
}
}
expect(store.getId()).to.equal(3)
store.createMany(toStore)
store.erase('id')
expect(store.fetch('id')).to.equal(undefined)
store.eraseMany('birth', 'attributes');
expect(store.all()).to.deep.equal({name: 'Jose'})
const store = require('@byu-oit/node-global-data-manager').Manager()
const { expect } = require('chai')
store.put('id', 1)
const res = store.copy('id', 'count')
expect(res).to.deep.equal({count: 1})
expect(store.fetch('count')).to.equal(1)
expect(store.fetch('id')).to.equal(1)
const store = require('@byu-oit/node-global-data-manager').Manager()
const { expect } = require('chai')
store.put('id', 1)
const res = store.rename('id', 'count')
expect(res).to.deep.equal({count: 1})
expect(store.fetch('count')).to.equal(1)
expect(store.fetch('id')).to.equal(undefined)
const store = require('@byu-oit/node-global-data-manager').Manager()
const { expect } = require('chai')
store.put('id', 1)
store.reset()
expect(store.all()).to.deep.equal({})
You can either set a ttl while using the put, putMany, create, or createMany methods
const store = require('@byu-oit/node-global-data-manager').Manager()
const { expect } = require('chai')
const duration = 3000
const offset = 1
const key = 'color'
const value = 'green'
store.put(key, value, duration)
clock.tick(duration - offset)
expect(store.fetch(key)).to.equal(value)
clock.tick(offset)
expect(store.fetch(key)).to.equal(undefined)
or set a ttl using the expire, or expireMany methods
const store = require('@byu-oit/node-global-data-manager').Manager()
const { expect } = require('chai')
const duration = 3000
const offset = 1
const key = 'color'
const value = 'green'
store.put(key, value)
store.expire(duration, key)
clock.tick(duration - offset)
expect(store.fetch(key)).to.equal(value)
clock.tick(offset)
expect(store.fetch(key)).to.equal(undefined)
const Manager = require('@byu-oit/node-global-data-manager')
const { expect } = require('chai')
const id = 'The Greatest Storage Ever'
const myStorage = Manager(id)
expect(myStorage.getId()).to.equal(id)
FAQs
Local server storage for quick caching
The npm package @byu-oit/node-global-data-manager receives a total of 47 weekly downloads. As such, @byu-oit/node-global-data-manager popularity was classified as not popular.
We found that @byu-oit/node-global-data-manager demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 12 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.