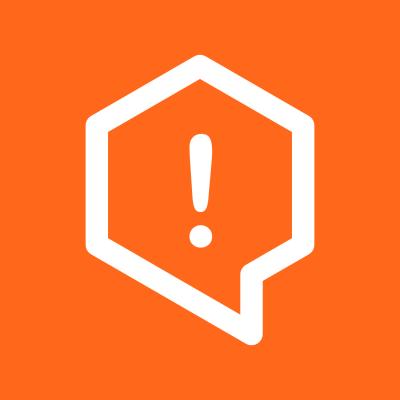
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
@castleio/castle-js
Advanced tools
Packaged version of Castle fingerprinting script.
The Castle JavaScript automatically captures every user action in your web application, including clicks, taps, swipes, form submissions, and page views. We use this data to build profiles of good user behavior in order to detect the bad.
npm install --save @castleio/castle-js
yarn add @castleio/castle-js
// @option options [string] :pk castle publishable key.
// @option options [object] :window (default `window`) eg JSDOM.window
// @option options [string] :apiUrl (default `https://m.castle.io/v1/monitor`) castle api url
// @option options [number] :timeout (default 1000) castle api response timeout
// @option options [boolean] :verbose (default true) verbose mode (console warnings)
// @option options [object] :storage (default name `__cuid`, default expireIn 400 days in seconds: `34560000`) used for storing uuid in the localStorage and the cookies, and for setting cookie expiration time.
import * as Castle from '@castleio/castle-js'
Castle.configure(options);
If your environment does not support modules you can use the browser version
import '@castleio/castle-js/dist/castle.browser.js'
Castle.configure(options);
import * as Castle from '@castleio/castle-js'
Castle.createRequestToken().then( (requestToken) => {
....
});
// or
const requestToken = await Castle.createRequestToken();
See Castle Docs for more information and how to pass the token further.
// @param event [Event] submit event
// @param onDone [Function] optional form submit callback replacement
<form action="/" onsubmit="Castle.injectTokenOnSubmit(event)">
<button type="submit">Submit</button>
</form>
helper is also available directly (when castle.umd.js is used)
import * as Castle from '@castleio/castle-js'
const submitHandler = (evt) => Castle.injectTokenOnSubmit(evt);
<form action="/" onsubmit="submitHandler(event)">
<button type="submit">Submit</button>
</form>
// @param options [PageParams] castle page command params.
// @option user [UserParams] user object with the required `id`, optional `email`, `phone`, `registered_at`, `name`, `traits`
// @option userJwt [string] optiona jwt encoded UserParams
// @option name [String]
// @option referrer [String]
// @option url [String]
import * as Castle from '@castleio/castle-js'
const page = Castle.page(options);
event response can be verified with promise like call.
import * as Castle from '@castleio/castle-js'
// result - true - success response from the server
// result - false - error response from the server, missing configuration or data
// result - null - timeout or debounced event (300ms)
Castle.page(options).then( (result) => { } )
// @param options [FormParams] castle form command params.
// @option user [UserParams] user object with the required `id`, optional `email`, `phone`, `registered_at`, `name`, `traits`
// @option userJwt [string] JWT encoded UserParams (can be passed instead of the user)
// @option name [String]
// @option values [Record<string, string>]
import * as Castle from '@castleio/castle-js'
Castle.form(options);
event response can be verified with promise like call.
import * as Castle from '@castleio/castle-js'
// result - true - success response from the server
// result - false - error response from the server, missing configuration or data
// result - null - timeout or throttled event (300ms)
Castle.form(options).then( (result) => { } )
import * as Castle from '@castleio/castle-js'
const user = YOUR_USER_DATA;
const submitHandler = (evt) => Castle.formEventOnSubmit(evt, user);
<form action="/" data-castle-name="Change Profile" onsubmit="submitHandler(event)">
<input type="text" name="user_mail" data-castle-value="email">
<button type="submit">Submit</button>
</form>
// @param options [CustomParams] castle custom command params.
// @option user [UserParams] user object with the required `id`, optional `email`, `phone`, `registered_at`, `name`, `traits`
// @option userJwt [string] JWT encoded UserParams (can be passed instead of the user)
// @option name [String]
// @option properties [Record<string,string>]
import * as Castle from '@castleio/castle-js'
const custom = Castle.custom(options);
event response can be verified with promise like call.
import * as Castle from '@castleio/castle-js'
// result - true - success response from the server
// result - false - error response from the server, missing configuration or data
// result - null - timeout or debounced event (default 1000ms)
Castle.custom(options).then( (result) => { } )
Legacy package: https://www.npmjs.com/package/castle.js
require "castle.js"
_castle('setAppId', "YOUR_APP_ID")
_castle('getClientId')
New package:
import * as Castle from "@castleio/castle-js"
_castle
to Castle
in the new module, but kept the _castle
in the CDN version as well as the browser-specific module @castleio/castle-js/dist/castle.browser.js
Castle.createRequestToken().then( (requestToken) => {
});
// or
const token = await Castle.createRequestToken();
autoForwardClientId
, autoTrack
, catchHistoryErrors
, identify
, setUserId
, setAccount
, setKey
, setAccount
, sessionId
, reset
, page
, trackPageView
, setTrackerUrl
Request Token is now required to be passed in the request params and it is no longer available in the cookie. Check the docs
Castle.configure(YOUR_CASTLE_APP_ID);
Removed the _castle
object for all versions and now only relying on Castle
.
Switched to use the Publishable Key that can be found in the same place as the now deprecated App ID.
Castle.configure({pk: YOUR_PUBLISHABLE_KEY});
onFormSubmit
has been renamed to injectTokenOnSubmit
_castle
global method is no longer supported and has been replaced with Castle
(for the CDN and castle.browser.js versions) check docs
CDN version no longer needs appID
in the url and requires
<script>Castle.configure({pk: YOUR_CASTLE_PUBLISHABLE_KEY});</script>
to be added. The CDN version can't be used for generating request tokens, but only for tracking client-side events.
page
, form
, and custom
methods for client-side event trackingMore info can be found in the docs
throttling
, enabled by default, and set to 500ms, is used when multiple custom events are being sent within short time intervals.storage
, deprecated storageNamespace
, and stability improvements.storageNamespace
, for specifing localStorage and cookie storage namespace, stability improvementscreateRequestToken()
is supported by the following browsers:
page()
, form()
and custom()
events are supported by the following browsers:
MIT
FAQs
Castle Fingerprinting Script
We found that @castleio/castle-js demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.