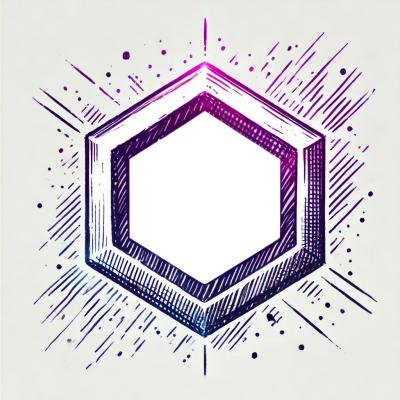
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
@chakra-ui/slider
Advanced tools
@chakra-ui/slider is a component library for creating highly customizable and accessible sliders in React applications. It is part of the Chakra UI library, which provides a set of accessible, reusable, and composable React components.
Basic Slider
This code demonstrates a basic slider with a default value of 30, a minimum value of 0, a maximum value of 100, and a step value of 5.
```jsx
import { Slider, SliderTrack, SliderFilledTrack, SliderThumb } from '@chakra-ui/react';
function BasicSlider() {
return (
<Slider defaultValue={30} min={0} max={100} step={5}>
<SliderTrack>
<SliderFilledTrack />
</SliderTrack>
<SliderThumb />
</Slider>
);
}
```
Vertical Slider
This code demonstrates a vertical slider with a default value of 30, a minimum value of 0, a maximum value of 100, and a step value of 5. The height of the slider is set to 200px.
```jsx
import { Slider, SliderTrack, SliderFilledTrack, SliderThumb } from '@chakra-ui/react';
function VerticalSlider() {
return (
<Slider orientation='vertical' defaultValue={30} min={0} max={100} step={5} height='200px'>
<SliderTrack>
<SliderFilledTrack />
</SliderTrack>
<SliderThumb />
</Slider>
);
}
```
Slider with Tooltip
This code demonstrates a slider with a tooltip that shows the current value of the slider. The tooltip appears when the user hovers over the slider thumb.
```jsx
import { Slider, SliderTrack, SliderFilledTrack, SliderThumb, Tooltip } from '@chakra-ui/react';
import { useState } from 'react';
function SliderWithTooltip() {
const [sliderValue, setSliderValue] = useState(30);
const [showTooltip, setShowTooltip] = useState(false);
return (
<Slider
id='slider'
defaultValue={30}
min={0}
max={100}
step={5}
onChange={(v) => setSliderValue(v)}
onMouseEnter={() => setShowTooltip(true)}
onMouseLeave={() => setShowTooltip(false)}
>
<SliderTrack>
<SliderFilledTrack />
</SliderTrack>
<Tooltip
hasArrow
bg='teal.500'
color='white'
placement='top'
isOpen={showTooltip}
label={`${sliderValue}%`}
>
<SliderThumb />
</Tooltip>
</Slider>
);
}
```
rc-slider is a React component for creating sliders. It is highly customizable and provides a range of features similar to @chakra-ui/slider, such as vertical orientation, tooltips, and custom styling. However, rc-slider does not come with built-in accessibility features like Chakra UI components.
react-slider is a small, fast, and customizable slider component for React. It supports horizontal and vertical orientations, custom styling, and range sliders. While it offers similar functionality to @chakra-ui/slider, it may require additional work to ensure accessibility.
material-ui-slider is part of the Material-UI library, which provides a set of React components that implement Google's Material Design. The slider component in Material-UI offers similar features to @chakra-ui/slider, including customization and accessibility, but follows Material Design guidelines.
The Slider is used to allow users to make selections from a range of values.
Sliders reflect a range of values along a bar, from which users may select a single value. They are ideal for adjusting settings such as volume, brightness, or applying image filters.
yarn add @chakra-ui/slider
# or
npm i @chakra-ui/slider
import {
Slider,
SliderTrack,
SliderFilledTrack,
SliderThumb,
} from "@chakra-ui/react"
<Slider defaultValue={30}>
<SliderTrack>
<SliderFilledTrack />
</SliderTrack>
<SliderThumb />
</Slider>
<Slider colorScheme="pink" defaultValue={30}>
<SliderTrack>
<SliderFilledTrack />
</SliderTrack>
<SliderThumb />
</Slider>
Slider component was designed to be composed to make it easy for you to customize its styles.
<Slider defaultValue={30}>
<SliderTrack bg="red.100">
<SliderFilledTrack bg="tomato" />
</SliderTrack>
<SliderThumb size={6}>
<Box color="tomato" as={MdGraphicEq} />
</SliderThumb>
</Slider>
FAQs
Accessible slider component for React that implements
We found that @chakra-ui/slider demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.