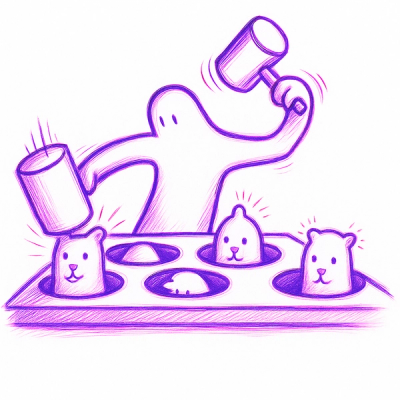
Research
/Security News
Contagious Interview Campaign Escalates With 67 Malicious npm Packages and New Malware Loader
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.
@chiragrupani/fullscreen-react
Advanced tools
Allows any html element to enter full screen using Browser API.
Install the package
npm i @chiragrupani/fullscreen-react
Use with class component or function component like below:
Class component example
<button onClick={e => this.setState({isFullScreen: true})}>Go Fullscreen</button>
<FullScreen
isFullScreen={this.state.isFullScreen}
onChange={(isFullScreen) => {
this.setState({ isFullScreen });
}}
>
<div>Fullscreen</div>
</FullScreen>
Function component example
export default function FSExampleHook() {
let [isFullScreen, setFullScreen] = React.useState(false);
return (
<>
<button onClick={(e) => setFullScreen(true)}>Go Fullscreen</button>
<FullScreen
isFullScreen={isFullScreen}
onChange={(isFull: boolean) => {
setFullScreen(isFull);
}}
>
<div>Fullscreen</div>
</FullScreen>
</>
);
}
If you require entire document in fullscreen instead of any specific element use DocumentFullScreen
instead of FullScreen
like below. However, there can be atmost one DocumentFullScreen:
<DocumentFullScreen
isFullScreen={this.state.isFullScreen}
onChange={(isFullScreen) => {
this.setState({ isFullScreen });
}}
>
<div>Fullscreen</div>
</DocumentFullScreen>
FAQs
Library to use full screen in ReactJS
The npm package @chiragrupani/fullscreen-react receives a total of 145 weekly downloads. As such, @chiragrupani/fullscreen-react popularity was classified as not popular.
We found that @chiragrupani/fullscreen-react demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.