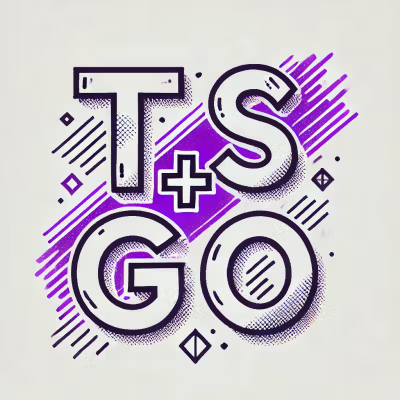
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
@codemask-labs/forms
Advanced tools
`codemask-labs/forms` is a continuation of old `react-form-builder-v2` package. Currenctly in **alpha** due to breaking changes and testing.
codemask-labs/forms
is a continuation of old react-form-builder-v2
package. Currenctly in alpha due to breaking changes and testing.
New features:
import z from 'zod'
const useOTPFormSchema = () => {
const phoneNumber = z
.string()
// chain your validation logic
.length(9, {
// override default zod validation messages
message: 'Phone number must have 9 digits'
})
.default('')
const otpCode = z
.string()
.optional() // mark field as optional
.default('123456') // set default values eg. when you want to prefill the form, must be called at the end of the chain
return {
phoneNumber,
otpCode
}
}
useForm
:const App = () => {
const { form, submit, isValid } = useForm({
fields: useOTPFormSchema(),
onSuccess: ({ phoneNumber, otpCode }) => {
// form is valid!
},
onError: errors => {
// form is invalid!
}
})
return (
<form
onSubmit={event => {
event.preventDefault()
submit()
}}
>
<Input field={form.name} />
<Input field={form.email} />
<button disabled={!isValid}>
Submit
</button>
</form>
)
}
field
to your form components:const Input: React.FunctionComponent<{ field: ReactiveFormField<string> }> = ({ field: useField }) => {
const { value, onChange, reset, error, validate } = useField()
return (
<div>
<input
value={value}
onChange={event => onChange(event.target.value)}
onBlur={() => validate()}
/>
<span>{error}</span>
<button
type='button'
onClick={reset}
>
Reset
</button>
</div>
)
}
[!WARNING]
If you use React Compiler, make sure you renamefield
touseField
- otherwise entire hook will be memoized and won't update the state
prop | type | functionality |
---|---|---|
form | Record<string, ReactiveFormField<any>> | all fields that should be passed to corresponding components |
submit | () => void | Submit the form |
reset | (...args?: Array<string>) => void | resets either entire form or specific fields |
getValues | () => Record<string, any> | Returns your form values as object |
isValid | boolean | Defines if your form is valid |
updateForm | (fields: Partial<Record<string, Partial<FormField<any>>>) => void | stanjs method to update form state |
useFormEffect | (fields: Record<string, any>) => void | stanjs effect - function that allows to subscribe to store's values change |
prop | type | functionality |
---|---|---|
fields | Record<string, ZodDefault<any>> | zod based schema for your form |
onSuccess | (fields: Record<string, any>) => void | called when your form is valid |
onError | (errors: Record<string, string>) => void | called when form is not valid, returns a pair for field key and error message |
onSubmit | (fields: Record<string, any>) => void | called after the validation, but before onSuccess or onError, useful for additonal validation like dependent fields |
prop | type | functionality |
---|---|---|
error | string | field error message passed from zod |
hasError | boolean | defines if your field has error |
value | any | current field value |
onChange | (value: any) => void | function to update current field value |
reset | () => void | resets current field to defaults |
validate | () => void | should be called to validate the field eg. onBlur event |
If you want to dynamically set field as optional you can either conditionally return different schemas, or create yourself simple util to do this:
export const dynamicOptional = <TSchema extends z.ZodDefault<z.ZodTypeAny>>(schema: TSchema, optional?: boolean) =>
optional ? schema.optional().default(schema._def.defaultValue()) : schema
FAQs
`codemask-labs/forms` is a continuation of old `react-form-builder-v2` package. Currenctly in **alpha** due to breaking changes and testing.
We found that @codemask-labs/forms demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.