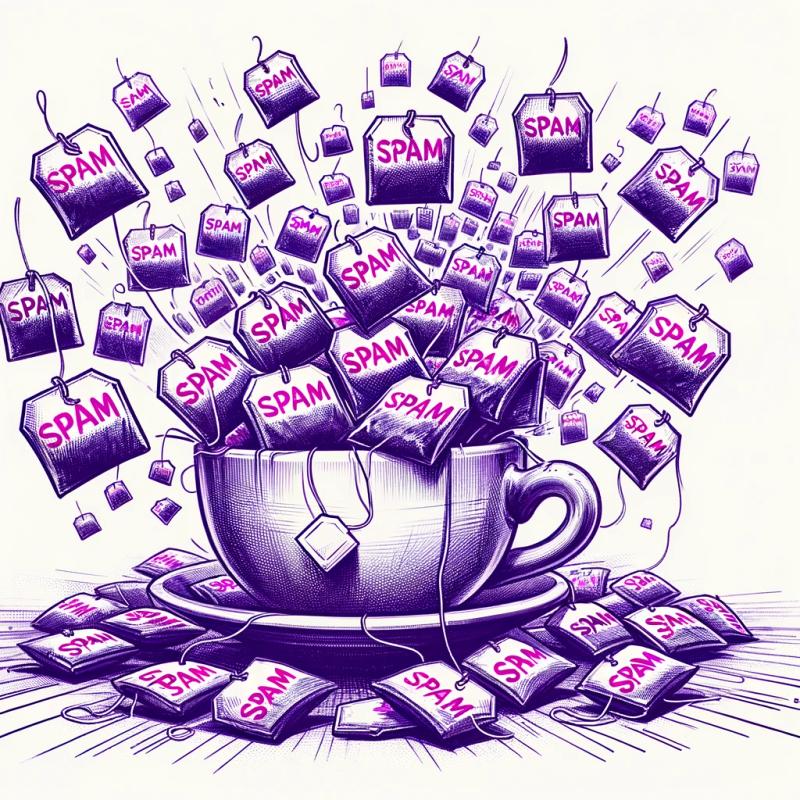
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@codesandbox/sandpack-react
Advanced tools
Readme
React components that give you the power of editable sandboxes that run in the browser.
import { Sandpack } from "@codesandbox/sandpack-react";
<Sandpack template="react" />;
For full documentation, visit https://sandpack.codesandbox.io/docs/
FAQs
Unknown package
The npm package @codesandbox/sandpack-react receives a total of 63,492 weekly downloads. As such, @codesandbox/sandpack-react popularity was classified as popular.
We found that @codesandbox/sandpack-react demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.