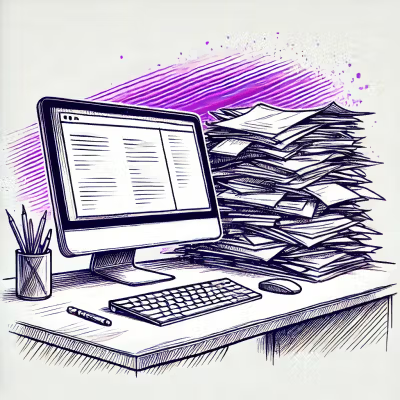
Security News
High Salaries No Longer Enough to Attract Top Cybersecurity Talent
A survey of 500 cybersecurity pros reveals high pay isn't enough—lack of growth and flexibility is driving attrition and risking organizational security.
@commodityvectors/react-mapbox-gl
Advanced tools
A simple and customizable MapboxGL wrapper for react
npm install @commodityvectors/react-mapbox-gl --save
Make sure you have all peers dependencies required installed on your project.
React Version | React Mapbox GL Version |
---|---|
16.3.x | 2.x.x |
16.2.x | 1.x.x |
Example:
import React from 'react';
import { Map } from '@commodityvectors/react-mapbox-gl';
export default class MyMap extends React.Component {
render() {
return (
<Map {...this.props} accessToken={"YOUR_ACCESS_TOKEN"} mapStyle={'mapbox://styles/mapbox/streets-v9'}>
{this.props.children}
</Map>
);
}
}
It is pretty simple to write your own map components. This library takes advantage of the new React 16.3 context API so you
can enrich you component with Map.component()
method like below. This helper will automatically add a MapContext.Provider
and inject the map as a prop
.
Example:
import React from 'react';
import mapboxgl from 'mapbox-gl';
import { Map } from '@commodityvectors/react-mapbox-gl';
class FullscreenControl extends React.Component {
componentDidMount() {
const { map } = this.props;
this.control = new mapboxgl.FullscreenControl();
map.addControl(this.control);
}
componentWillUnmount() {
const { map } = this.props;
if(!map.isRemoving()) map.removeControl(this.control);
}
render() {
return null; // As this component won't change through render methods
}
}
export default Map.component(FullscreenControl);
In order to extend an existing component, you have to propagate the props (or at least the map prop) to the child component as the example below shows
import React from 'react';
import { CustomControl, Map } from '@commodityvectors/react-mapbox-gl';
class Control extends React.PureComponent {
state = {
position: 'top-right'
};
recenter = () => {
this.props.map.easeTo({
center: [0, 0],
zoom: 1
});
};
render() {
const positions = {
'top-right': 'bottom-left',
'bottom-left': 'top-right'
};
return (
<CustomControl position={this.state.position} map={this.props.map}> {/* {...this.props} pass the map instance to the component below */}
<button style={{
'width': '120px',
'padding': '5px'
}} onClick={() => this.setState({position: positions[this.state.position]})}>Change Position</button>
<button style={{
'width': '120px',
'padding': '5px'
}} onClick={this.recenter}>Recenter</button>
</CustomControl>
);
}
}
export default Map.component(Control);
Property Name | Description | Type | Required |
---|---|---|---|
accessToken | Mapbox access token | string | YES |
mapStyle | The map 'style' property | object/string | YES |
bearing | The map 'bearing' property | number | |
pitch | The map 'pitch' property | number | |
zoom | The map 'zoom' property | number | |
center | The map 'center' property | object/Array | |
options | Every other map property | object | |
onViewPortChange | Called when bearing, pitch, center or zoom changes | function | |
mapNodeStyle | The map parent component style | object |
Property Name | Description | Type | Required |
---|---|---|---|
id | The layer id | string | YES |
type | The layer type | string | YES |
visible | Shortcut to set paint property "visibility" | boolean | |
before | The ID of an existing layer to add thi one before | string | |
options | Every other layer properties, you can use cameCase | object |
Property Name | Description | Type | Required |
---|---|---|---|
id | The layer id | string | YES |
type | Layer type (as found on mapbox documentation) | string | YES |
options | Every other source properties | object | YES |
Property Name | Description | Type | Required |
---|---|---|---|
position | This popup coordinates | Array/object | YES |
onClose | Called when popup is closed | function | |
options | Every other popup properties | object |
Property Name | Description | Type | Required |
---|---|---|---|
position | This marker coordinates | Array/object | YES |
onClick | Called when marker is clicked | function | |
data | Data to be sent in click event | any |
Property Name | Description | Type | Required |
---|---|---|---|
position | This control position | string | |
options | Every other properties | object |
Property Name | Description | Type | Required |
---|---|---|---|
position | This control position | string | |
options | Every other properties | object |
Property Name | Description | Type | Required |
---|---|---|---|
position | This control position | string | |
options | Every other properties | object |
Property Name | Description | Type | Required |
---|---|---|---|
position | This control position | string | |
options | Every other properties | object |
Property Name | Description | Type | Required |
---|---|---|---|
position | This control position | string |
Property Name | Description | Type | Required |
---|---|---|---|
position | This control position (top-right, bottom-left,...) | string |
FAQs
A minimal React MapboxGL component
The npm package @commodityvectors/react-mapbox-gl receives a total of 91 weekly downloads. As such, @commodityvectors/react-mapbox-gl popularity was classified as not popular.
We found that @commodityvectors/react-mapbox-gl demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A survey of 500 cybersecurity pros reveals high pay isn't enough—lack of growth and flexibility is driving attrition and risking organizational security.
Product
Socket, the leader in open source security, is now available on Google Cloud Marketplace for simplified procurement and enhanced protection against supply chain attacks.
Security News
Corepack will be phased out from future Node.js releases following a TSC vote.