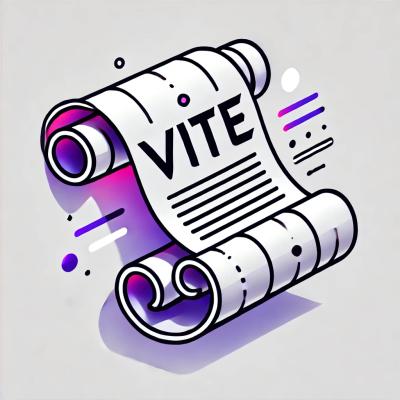
Security News
Vite Releases Technical Preview of Rolldown-Vite, a Rust-Based Bundler
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
@cornerstonejs/codec-charls
Advanced tools
JS/WebAssembly build of CharLS
Try it in your browser here
Install this in your JavaScript project using npm:
# NOTE - this is not published yet so won't work yet...
#npm install --save-dev charls-js
Before using this library, you must wait for it to be initialized:
const charls = require('charlsjs')
charls.onRuntimeInitialized = async _ => {
// Now you can use it
}
To decode a JPEG-LS image, create a decoder instance, copy the JPEG-LS bitstream into its memory space, decode it, copy the decoded pixels out of its memory space and finally, delete the decoder instance.
function decode(jpeglsEncodedBitStream) {
// Create a decoder instance
const decoder = new charls.JpegLSDecoder();
// get pointer to the source/encoded bit stream buffer in WASM memory
// that can hold the encoded bitstream
const encodedBufferInWASM = decoder.getEncodedBuffer(jpeglsEncodedBitStream.length);
// copy the encoded bitstream into WASM memory buffer
encodedBufferInWASM.set(jpeglsEncodedBitStream);
// decode it
decoder.decode();
// get information about the decoded image
const frameInfo = decoder.getFrameInfo();
const interleaveMode = decoder.getInterleaveMode();
const nearLossless = decoder.getNearLossless();
// get the decoded pixels
const decodedPixelsInWASM = decoder.getDecodedBuffer();
// TODO: do something with the decoded pixels here (e.g. display them)
// The pixel arrangement for color images varies depending upon the
// interleaveMode parameter, see documentation in JpegLSDecode::getInterleaveMode()
// delete the instance. Note that this frees up memory including the
// encodedBufferInWASM and decodedPixelsInWASM invalidating them.
// Do not use either after calling delete!
decoder.delete();
}
const jpeglsEncodedBitStream = // read from file, load from URL, etc
decode(jpeglsEncodedBitStream)
See examples for browsers and nodejs. Also read the API documentation for JpegLSDecoder.hpp and JpegLSEncoder.hpp
See information about building here
Read about the design considerations that went into this library here
Read about the encode/decode performance of this library with NodeJS 14, Google Chrome and FireFox vs Native here
FAQs
WASM Build of CharLS
We found that @cornerstonejs/codec-charls demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
Research
Security News
A malicious npm typosquat uses remote commands to silently delete entire project directories after a single mistyped install.
Research
Security News
Malicious PyPI package semantic-types steals Solana private keys via transitive dependency installs using monkey patching and blockchain exfiltration.