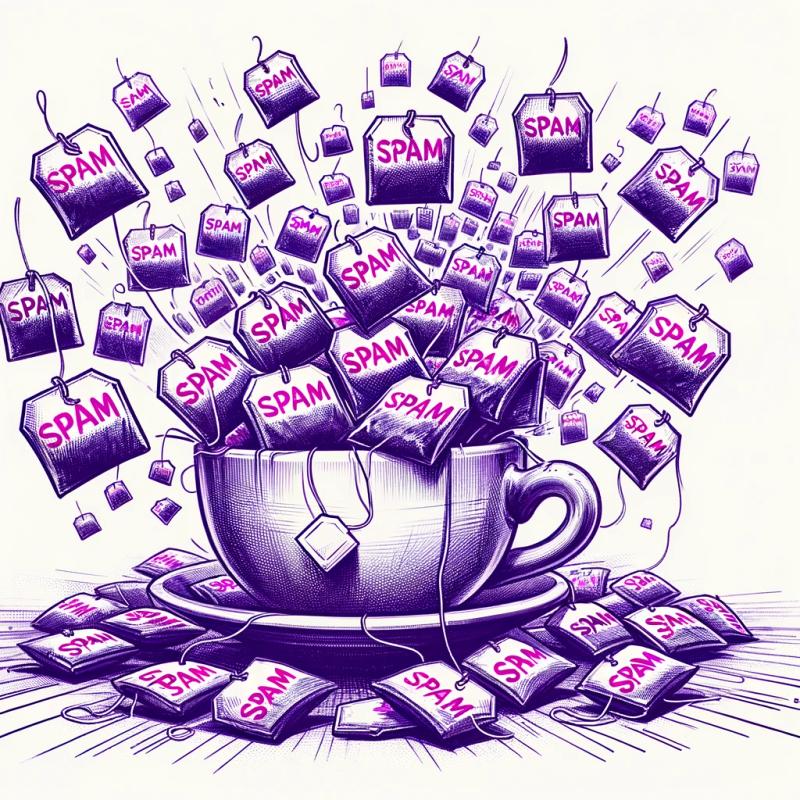
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@dolbyio/webrtc-stats
Advanced tools
Readme
This project is a library to use to parse WebRTC statistics.
Run the npm command to install the package @dolbyio/webrtc-stats
into your project:
npm install @dolbyio/webrtc-stats --save
A WebRTCStats
object needs to be created to start a WebRTC statistics collection. It requires some settings to configure how you want the collection to work.
interface WebRTCStatsOptions {
/**
* Function that will be called to retrieve the WebRTC statistics.
* @returns a {@link RTCStatsReport} object through a {@link Promise}.
*/
getStats: () => Promise<RTCStatsReport>;
/**
* Interval, in milliseconds, at which to collect the WebRTC statistics.
* Default is 1,000 ms (1 second).
*/
getStatsInterval?: number;
/**
* Include the raw statistics in the `stats` event.
* Default is `false`.
*/
includeRawStats?: boolean;
}
Create the collection object.
const collection = new WebRTCStats({
getStats: () => {
// Get the raw WebRTC statistics from the web browser
},
getStatsInterval: 1000,
includeRawStats: true,
});
Start the collection with the start()
function.
collection.start();
Stop the collection with the stop()
function.
collection.stop();
After starting the collection, the stats
event is triggered when the WebRTC statistics have been collected and parsed. The event
object is of type OnStats.
collection.on('stats', (event) => {
console.log(event);
});
The error
event is triggered when an error happens during the collection or the parsing of the WebRTC statistics.
collection.on('error', (error) => {
console.error(error);
});
Example on how to start a statistics collection from the Dolby.io Real-time Streaming APIs.
import WebRTCStats from '@dolbyio/webrtc-stats';
import { Director, Publish } from '@millicast/sdk';
const PUBLISHER_TOKEN = '';
const STREAM_NAME = '';
const tokenGenerator = () =>
Director.getPublisher({
token: PUBLISHER_TOKEN,
streamName: STREAM_NAME,
});
const publisher = new Publish(STREAM_NAME, tokenGenerator);
// Publish the stream
const collection = new WebRTCStats({
getStatsInterval: 1000,
getStats: () => {
return publisher.webRTCPeer.getRTCPeer().getStats();
},
});
// The stats event is triggered after each interval has elapsed
collection.on('stats', (event) => {
// Triggered when the statistics have been parsed
console.log(event);
});
// Start the statistics collection
collection.start();
Example on how to start a statistics collection from the Dolby.io Communications APIs.
import WebRTCStats from '@dolbyio/webrtc-stats';
import VoxeetSdk from '@voxeet/voxeet-web-sdk';
const collection = new WebRTCStats({
getStatsInterval: 1000,
getStats: async () => {
// See: https://docs.dolby.io/communications-apis/docs/js-client-sdk-conferenceservice#localstats
const webRTCStats = await VoxeetSDK.conference.localStats();
// Convert the WebRTCStats object to RTCStatsReport
const values = Array.from(webRTCStats.values())[0];
const map = new Map();
for (let i = 0; i < values.length; i++) {
const element = values[i];
map.set(element.id, element);
}
return map;
},
});
// The stats event is triggered after each interval has elapsed
collection.on('stats', (event) => {
// Triggered when the statistics have been parsed
console.log(event);
});
// Start the statistics collection
collection.start();
Run tests:
npm run test
Create distribution package:
npm run build
FAQs
Dolby.io WebRTC Statistics
The npm package @dolbyio/webrtc-stats receives a total of 224 weekly downloads. As such, @dolbyio/webrtc-stats popularity was classified as not popular.
We found that @dolbyio/webrtc-stats demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.