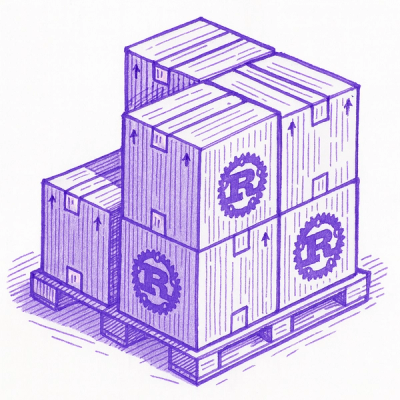
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
@dydxprotocol/dydx.js
Advanced tools
A TypeScript library for interacting with the dYdX protocol.
npm install --save @dydxprotocol/dydx.js
import { DYDX } from '@dydxprotocol/dydx.js';
const dydx = new DYDX();
await dydx.initialize(provider, networkId);
await dydx.shortToken.mint(
positionId,
trader,
tokensToMint,
payInHeldToken,
exchangeWrapper,
orderData,
options,
);
Or mint with ETH:
await dydx.shortToken.mintWithETH(
positionId,
trader,
tokensToMint,
ethToSend,
ethIsHeldToken,
exchangeWrapper,
orderData,
options,
);
Or mint directly (you will put up all held token [DAI for sETH] and will receive owed token [WETH for sETH]):
// Set your allowance on our proxy contract - you only need to do this once
await dydx.token.setMaximumProxyAllowance(
heldTokenAddress, // DAI address for sETH
traderAddress, // your address
);
await dydx.shortToken.mintDirectly(
positionId, // Can get from expo API
trader, // your address
tokensToMint, // BigNumber - Number of tokens to mint in base units (10^18 is 1 sETH)
);
Close directly (you will pay all owed token owed to lenders [WETH for sETH] and will receive all held token collateral [DAI for sETH])
// Set your allowance on our proxy contract - you only need to do this once
await dydx.token.setMaximumProxyAllowance(
owedTokenAddress, // WETH address for sETH
traderAddress, // your address
);
await dydx.shortToken.closeDirectly(
positionId, // Can get from expo API
closer, // your address
tokensToClose, // BigNumber - Number of tokens to close in base units (10^18 is 1 sETH)
);
npm install
npm run build
FAQs
Javascript library for interacting with the dYdX Protocol
We found that @dydxprotocol/dydx.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.