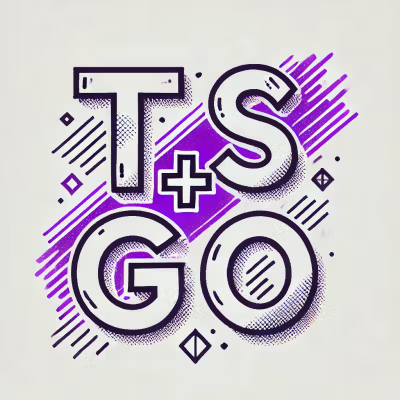
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
@egodigital/microservices
Advanced tools
Shared library for microservices, written for Node.js, in TypeScript.
Execute the following command from your project folder, where your package.json
file is stored:
npm install --save @egodigital/microservices
Hash passwords with bcrypt:
import { checkPassword, checkPasswordSync, hashPassword, hashPasswordSync } from '@egodigital/microservices';
const hash1 = await hashPassword('test');
const doesMatch1 = await checkPassword('test', hash1); // true
const hash2 = hashPasswordSync('test');
const doesMatch2 = checkPasswordSync('Test', hash2); // false
Sign and verify JSON Web Tokens:
import { signJWT, verifyJWT } from '@egodigital/microservices';
interface IUserToken {
uuid: string;
}
const jwt = signJWT({
uuid: 'cb246b52-b8cd-4916-bfad-6bfc43845597'
});
const decodedToken = verifyJWT<IUserToken>(jwt);
Use predefined Express middleware to verify and decode JWTs:
import express from 'express';
import { withJWT } from '@egodigital/microservices';
const app = express();
app.get('/', withJWT(), async (request, response) => {
// decoded, valid user token is stored in:
// request.userToken
});
app.listen(4242, () => {
console.log('Service is listening ...');
});
Name | Description | Example |
---|---|---|
BCRYPT_ROUNDS | The number of rounds for bcrypt hashing. Default: 10 | 12 |
JWT_SECRET | The secret for signing and validating JWT. | mySecretJWTSecret |
NATS_CLUSTER_ID | The name of the cluster, that contains all microservices. | my-cluster |
NATS_GROUP | The name of the pod group / Kubernetes deployment. | my-service-or-deployment |
NATS_URL | The URL to the NATS server. | http://my-nats-service:4222 |
POD_NAME | The name of the pod. This should come as imported metadata from Kubernetes. | my-service-or-deployment-xcsgbxv |
Connect to a NATS server:
import { stan } from '@egodigital/microservices';
await stan.connect();
stan.exitOnClose();
Listen for events:
import { NatsListener } from '@egodigital/microservices';
interface IMyEvent {
foo: string;
bar: number;
}
const myEventListener = new NatsListener<IMyEvent>('my.event');
myEventListener.onMessage = async (context) => {
// handle message in context.message of type IMyEvent
};
myEventListener.listen();
Publish events:
import { NatsPublisher } from '@egodigital/microservices';
interface IMyEvent {
foo: string;
bar: number;
}
const myEventPublisher = new NatsPublisher<IMyEvent>('my.event');
await myEventPublisher.publish({
foo: "TM+MK",
bar: 42
});
The API documentation can be found here.
0.7.0
npm update
FAQs
Shared library for microservices, written for Node.js
The npm package @egodigital/microservices receives a total of 1 weekly downloads. As such, @egodigital/microservices popularity was classified as not popular.
We found that @egodigital/microservices demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.