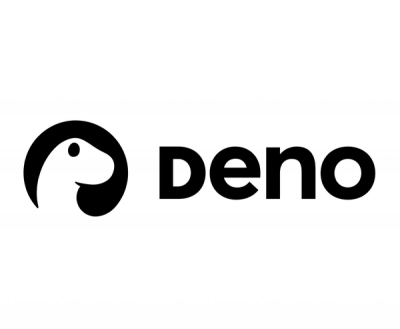
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@flexshopper/hapi-pres
Advanced tools
Plugin to autoload pres based on patterns.
hapi-pres
npm package in your project our plugin.
npm i @flexshopper/hapi-pres
Kraken service style:
const server = new Hapi.Server();
server.connection();
server.register({
register: require('hapi-routes'),
options: {
relativeTo: proccess.cwd() + '/pres',
includes: ['path/to/**/*pres.js'],
ignore: ['*.git'],
}
}, (err) => {
// continue application
});
Manifest style:
registrations: [
...
{
plugin: {
register: 'hapi-pres',
options: {
relativeTo: proccess.cwd() + '/pres',
includes: ['path/to/**/*pres.js'],
ignore: ['*.git'],
}
}
}
];
Required
Type: array
The glob pattern you would like to include
Type: array
The pattern or an array of patterns to exclude
Type: string
The current working directory in which to search (defaults to process.cwd()
)
const internals = module.exports = {};
internals.assign = 'myAwesomePre';
internals.method = (request, reply) => {
return reply(request.query);
};
// then in the routes
server.route({
method: 'GET',
path: '/ping',
config: {
pre: [
server.pre.myAwesomePre
],
handler: (request, reply) => {
return reply(request.pre.example);
}
}
});
// in you pre-handler file
const internals = module.exports = {};
internals.myAwesomePre = (request, reply) => {
return reply('myAwesomePre');
}
internals.mySecondAwesomePre = (request, reply) => {
return reply('mySecondAwesomePre');
}
// assume your pre-handler file name is my-Pre.js
// then in the routes
server.route({
method: 'GET',
path: '/ping',
config: {
pre: [
server.pre.myPre.myAwesomePre,
server.pre.myPre.mySecondAwesomePre
],
handler: (request, reply) => {
return reply('handler');
}
}
});
// if you need to get result from pre handler
request.pre.myAwesomePre
request.pre.mySecondAwesomePre
FAQs
Hapi plugin to autoload pres.
The npm package @flexshopper/hapi-pres receives a total of 31 weekly downloads. As such, @flexshopper/hapi-pres popularity was classified as not popular.
We found that @flexshopper/hapi-pres demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.